ammo.js 软体模拟例子
时间: 2023-08-18 18:07:22 浏览: 243
你可以使用 ammo.js 实现软体模拟。以下是一个简单的例子,展示了如何使用 ammo.js 创建一个软体模拟:
```javascript
// 引入 ammo.js
import * as Ammo from 'ammo.js';
// 创建 ammo.js 的世界
const collisionConfiguration = new Ammo.btDefaultCollisionConfiguration();
const dispatcher = new Ammo.btCollisionDispatcher(collisionConfiguration);
const broadphase = new Ammo.btDbvtBroadphase();
const solver = new Ammo.btSequentialImpulseConstraintSolver();
const world = new Ammo.btSoftRigidDynamicsWorld(dispatcher, broadphase, solver, collisionConfiguration);
world.setGravity(new Ammo.btVector3(0, -9.8, 0));
// 创建地面
const groundShape = new Ammo.btBoxShape(new Ammo.btVector3(50, 1, 50));
const groundTransform = new Ammo.btTransform();
groundTransform.setIdentity();
groundTransform.setOrigin(new Ammo.btVector3(0, -1, 0));
const groundMass = 0;
const groundLocalInertia = new Ammo.btVector3(0, 0, 0);
const groundMotionState = new Ammo.btDefaultMotionState(groundTransform);
const groundRigidBodyInfo = new Ammo.btRigidBodyConstructionInfo(groundMass, groundMotionState, groundShape, groundLocalInertia);
const groundRigidBody = new Ammo.btRigidBody(groundRigidBodyInfo);
world.addRigidBody(groundRigidBody);
// 创建软体
const softBodyWorldInfo = new Ammo.btSoftBodyWorldInfo();
const softBodySolver = new Ammo.btDefaultSoftBodySolver();
softBodyWorldInfo.air_density = 1.2;
softBodyWorldInfo.water_density = 0;
softBodyWorldInfo.water_offset = 0;
softBodyWorldInfo.water_normal = new Ammo.btVector3(0, 0, 0);
softBodyWorldInfo.m_gravity.setValue(0, -9.8, 0);
softBodyWorldInfo.m_sparsesdf.Initialize();
const clothCorner00 = new Ammo.btVector3(-5, 5, 0);
const clothCorner01 = new Ammo.btVector3(5, 5, 0);
const clothCorner10 = new Ammo.btVector3(-5, -5, 0);
const clothCorner11 = new Ammo.btVector3(5, -5, 0);
const clothSoftBody = Ammo.btSoftBodyHelpers.CreatePatch(softBodyWorldInfo, clothCorner00, clothCorner01, clothCorner10, clothCorner11, 10, 10, 0, true);
clothSoftBody.getCollisionShape().setMargin(0.5);
clothSoftBody.setTotalMass(0.1, false);
world.addSoftBody(clothSoftBody);
// 更新模拟
function update() {
world.stepSimulation(1 / 60, 10);
// 更新软体的位置
const softBodyNodes = clothSoftBody.get_m_nodes();
const nodeCount = softBodyNodes.size();
for (let i = 0; i < nodeCount; i++) {
const node = softBodyNodes.at(i);
const position = node.get_m_x();
console.log(`Node ${i}: x=${position.x()}, y=${position.y()}, z=${position.z()}`);
}
}
// 每帧更新
function animate() {
requestAnimationFrame(animate);
update();
}
animate();
```
这个例子创建了一个简单的软体模拟,包括一个地面和一个布料。你可以根据自己的需求进行修改和扩展。注意,这个例子是使用 ES6 模块语法,你需要使用支持模块的构建工具或浏览器环境来运行它。
阅读全文
相关推荐
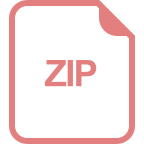


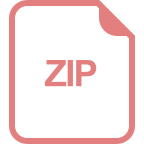
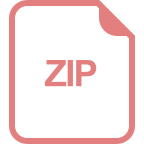
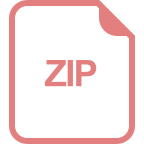
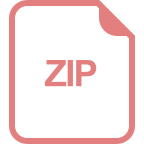
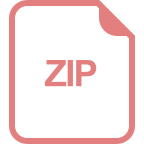
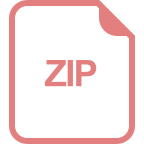
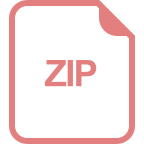
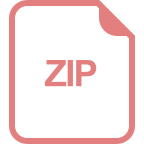
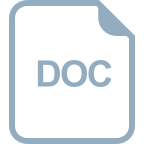




