编写c++代码,让用户输入一段链表,再使用反转链表函数将链表反转,最后输出
时间: 2024-09-16 14:05:28 浏览: 60
下面是一个简单的 C++ 示例,演示了如何让用户输入链表,然后反转链表并输出结果:
首先,我们定义链表节点结构:
```cpp
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
```
接着,编写函数来获取用户的输入,并创建链表:
```cpp
ListNode* createLinkedList() {
string input;
getline(cin, input);
// 将输入字符串分割成整数列表
vector<int> values;
istringstream iss(input);
int value;
while (iss >> value) {
values.push_back(value);
}
// 创建链表
ListNode* head = NULL, *tail = NULL;
for (int i = 0; i < values.size(); ++i) {
ListNode* node = new ListNode(values[i]);
if (!head) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
```
然后,定义一个反转链表的函数:
```cpp
ListNode* reverseList(ListNode* head) {
ListNode* prev = nullptr;
ListNode* current = head;
ListNode* next = nullptr;
while (current != nullptr) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev;
}
```
最后,反转链表并输出:
```cpp
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != nullptr) {
cout << temp->val << " ";
temp = temp->next;
}
cout << endl;
}
int main() {
ListNode* inputList = createLinkedList();
ListNode* reversedList = reverseList(inputList);
cout << "Original list: ";
printList(inputList);
cout << "Reversed list: ";
printList(reversedList);
delete inputList; // 释放内存
return 0;
}
```
当你运行这段代码时,它会提示用户输入链表,然后反转链表并在屏幕上显示原始链表和反转后的链表。
阅读全文
相关推荐
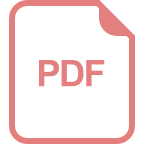
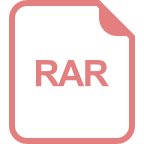
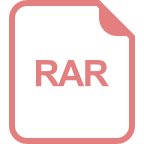
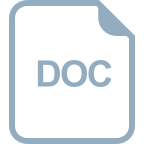
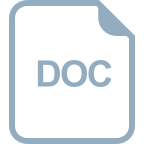
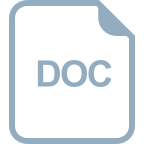












