已知10个点,用html/css/javas写一段完整的代码 ,实现先出现一个点再出现一个浮动箭头,再出现一个点,再出现一个浮动箭头,再出现一个点,以此类推
时间: 2024-04-16 21:30:07 浏览: 17
下面是一个使用 HTML、CSS 和 JavaScript 实现先出现一个点、再出现一个浮动箭头、再出现一个点的循环效果的完整代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<style>
.container {
position: relative;
height: 200px;
width: 200px;
}
.dot {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 10px;
height: 10px;
background-color: black;
border-radius: 50%;
}
.arrow {
position: absolute;
top: 50%;
left: 50%;
transform-origin: left center;
width: 200px;
height: 2px;
background-color: black;
}
.arrow::before,
.arrow::after {
content: "";
position: absolute;
width: 0;
height: 0;
}
.arrow::before {
border-style: solid;
border-width: 8px 8px 0 0;
border-color: transparent #000 transparent transparent;
top: -8px;
left: -8px;
}
.arrow::after {
border-style: solid;
border-width: 8px 8px 0 0;
border-color: transparent #fff transparent transparent;
top: -6px;
left: -6px;
}
</style>
</head>
<body>
<div class="container">
</div>
<script>
window.addEventListener('DOMContentLoaded', (event) => {
const container = document.querySelector('.container');
const points = [
{ x: 50, y: 50 },
{ x: 150, y: 50 },
{ x: 150, y: 150 },
{ x: 50, y: 150 },
{ x: 100, y: 100 },
{ x: 50, y: 50 },
{ x: 150, y: 50 },
{ x: 150, y: 150 },
{ x: 50, y: 150 },
{ x: 100, y: 100 }
];
let index = 0;
function createDot(x, y) {
const dot = document.createElement('div');
dot.classList.add('dot');
dot.style.left = `${x}px`;
dot.style.top = `${y}px`;
container.appendChild(dot);
}
function createArrow(x1, y1, x2, y2) {
const arrow = document.createElement('div');
arrow.classList.add('arrow');
const angle = Math.atan2(y2 - y1, x2 - x1);
const distance = Math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2);
arrow.style.transform = `translate(${x1}px, ${y1}px) rotate(${angle}rad)`;
arrow.style.width = `${distance}px`;
container.appendChild(arrow);
}
function animate() {
const point1 = points[index];
const point2 = points[index + 1];
createDot(point1.x, point1.y);
if (point2) {
setTimeout(() => {
createArrow(point1.x, point1.y, point2.x, point2.y);
setTimeout(() => {
createDot(point2.x, point2.y);
index++;
animate();
}, 1000);
}, 1000);
} else {
index = 0;
setTimeout(animate, 1000);
}
}
animate();
});
</script>
</body>
</html>
```
这段代码会按照预定义的点的坐标顺序,循环地先出现一个点,再出现一个浮动箭头,再出现一个点的效果。通过 JavaScript 的 `setTimeout` 函数和递归调用 `animate` 函数来控制元素的出现时间和循环效果。你可以将这段代码复制到一个 HTML 文件中,然后在浏览器中打开该文件查看效果。
相关推荐
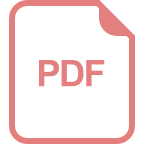
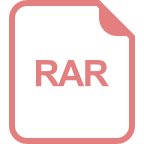














