unordered_map 的key为结构体
时间: 2023-10-15 12:29:10 浏览: 92
unordered_map 的 key 可以是结构体,但是需要满足以下条件:
1. 结构体必须实现自定义的哈希函数和相等运算符。因为 unordered_map 使用哈希表来实现,所以需要通过哈希函数将结构体映射为一个整数值,并且通过相等运算符来比较两个结构体是否相等。
2. 结构体的哈希函数应该将结构体的每个成员都考虑进去,以确保不同的结构体对应不同的哈希值。相等运算符应该比较结构体的每个成员是否相等。
以下是一个示例代码,演示了如何定义一个结构体作为 unordered_map 的 key:
```cpp
struct MyKey {
int a;
std::string b;
// 其他成员...
// 自定义哈希函数
size_t operator()(const MyKey& key) const {
size_t hash = std::hash<int>()(key.a);
hash_combine(hash, std::hash<std::string>()(key.b));
// 对其他成员进行哈希运算...
return hash;
}
// 自定义相等运算符
bool operator==(const MyKey& other) const {
return a == other.a && b == other.b /*&& 其他成员相等...*/;
}
// 辅助函数,用于合并哈希值
static void hash_combine(size_t& seed, const size_t& value) {
seed ^= value + 0x9e3779b9 + (seed << 6) + (seed >> 2);
}
};
std::unordered_map<MyKey, int> myMap;
```
注意,这只是一个简单示例,实际使用时可能需要根据结构体的具体成员和需求进行适当的修改。
阅读全文
相关推荐
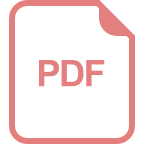



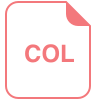
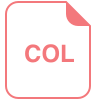
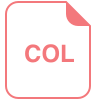
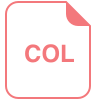









