#include<bits/stdc++.h> using namespace std; int main(){ string s; getline(cin,s); stringstream ss(s); string str; vector<string> nums; while(getline(ss,str,',')){ nums.push_back(str); } int m; cin>>m; vector<int> line; vector<vector<int>> lines; string strr; int len = nums.size(); for(int i=0; i<len; i++){ line.clear(); stringstream ss2(nums[i]); while(getline(ss2,strr,'-')){ line.push_back(stoi(strr)); } lines.push_back(line); } sort(lines.begin(), lines.end(), [](const vector<int>& a, const vector<int>& b){ return a[0] < b[0]; }); string ans1, ans2,ans3; for(auto& line : lines){ if(line.size() == 1){ if(line[0] == m){ nums.erase(remove(nums.begin(), nums.end(), to_string(line[0])), nums.end()); } } else if(line.size() == 2){ int l = line[0]; int r = line[1]; if(l < m && m < r){ ans3=to_string(l)+"-"+to_string(r); ans1 = to_string(l) + "-" + to_string(m - 1); ans2 = to_string(m + 1) + "-" + to_string(r); if(l == m - 1){ ans1 = to_string(l); } else if(r == m + 1){ ans2 = to_string(r); } } } } if(!ans1.empty()){ nums.push_back(ans1); } if(!ans2.empty()){ nums.push_back(ans2); } nums.erase(remove_if(nums.begin(), nums.end(), [](const string& num){ return num == ans3; }), nums.end()); for(int i=0; i<nums.size(); i++){ cout << nums[i]; if(i != nums.size() - 1){ cout << ","; } } return 0; }C:\Program Files\CodeBlocks\bei\main.cpp|57|error: 'ans3' is not captured|
时间: 2024-04-16 08:29:03 浏览: 113
在你的代码中,出现了一个错误,指示'ans3'未被捕获。这是因为你在lambda函数中引用了'ans3'变量,但未在函数签名中进行捕获。
要解决这个问题,你可以简单地通过在lambda函数的方括号中添加'ans3'来捕获它,如下所示:
```cpp
nums.erase(remove_if(nums.begin(), nums.end(), [ans3](const string& num){
return num == ans3;
}), nums.end());
```
通过这样做,你就可以在lambda函数中访问和使用'ans3'变量了。
请注意,在C++11中,lambda函数默认不捕获任何外部变量。如果你想在lambda函数中使用某个外部变量,你必须显式将其捕获。
相关问题
#include<bits/stdc++.h> using namespace std; int main() { string s; getline(cin,s); cout<<s<<endl; //cout<<s<<endl; int a[10000],i=0,j; stringstream stream(s); while(stream>>a[i++]); /**/ cout<<a[0];//是这个意思叭 fo
这段代码没有完成,最后的注释也没有写完整,无法判断出它的具体作用。但是可以看出代码使用了 stringstream 对象将输入的字符串转换成整型数组,然后输出数组中的第一个元素。
以下是这段代码的完整版本,它可以将输入的字符串保存到 stringstream 对象中,并将其中的整数存入整型数组中,最后输出数组中的第一个元素:
```cpp
#include <iostream>
#include <sstream>
using namespace std;
int main() {
string s;
getline(cin, s);
cout << s << endl;
int a[10000], i = 0;
stringstream stream(s);
while (stream >> a[i++]);
cout << a[0];
return 0;
}
```
输入示例:
```
1 2 3 4 5
```
输出示例:
```
1 2 3 4 5
1
```
#include <bits/stdc++.h> using namespace std; typedef struct{ string s; }tree[101]; int main() { int n; cin>>n; for(int i=1;i<=n;i++){ cin>>tree[i].s; getline(cin,s); } int a[101]={1}; for(int i=1;i<=n;i++){ for(int j=i+1;j<=n;j++){ if(str[j]==str[i]){ a[j]++; } } } for(int i=1;i<=n;i++){ cout<<a[i]<<endl; } return 0; }为什么不对呢
这段代码存在一些问题,导致编译错误。首先,你在定义结构体时使用了一个未定义的类型 `string`,应该包含头文件 `<string>` 来解决这个问题。另外,在输入循环中你使用了 `getline(cin, s)`,但是你并没有定义变量 `s`,应该改为 `getline(cin, tree[i].s)`。此外,比较字符串是否相等时,应该使用 `tree[j].s == tree[i].s` 而不是 `str[j] == str[i]`。最后,你输出的数组应该是 `a` 而不是 `str`。下面是修改后的代码:
```cpp
#include <bits/stdc++.h>
using namespace std;
typedef struct {
string s;
} tree[101];
int main()
{
int n;
cin >> n;
cin.ignore(); // 忽略换行符
for (int i = 1; i <= n; i++) {
getline(cin, tree[i].s);
}
int a[101] = { 0 }; // 初始化为0
for (int i = 1; i <= n; i++) {
for (int j = i + 1; j <= n; j++) {
if (tree[j].s == tree[i].s) {
a[j]++;
}
}
}
for (int i = 1; i <= n; i++) {
cout << a[i] << endl;
}
return 0;
}
```
这样修改后,代码应该可以正常编译和运行。
阅读全文
相关推荐
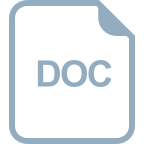
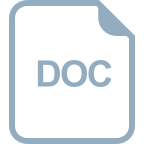
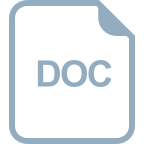













