请帮我测试下列代码是否能正常运行,并告知我下列代码的作用:class Trade: def __init__(self, symbol, quantity, price): self.symbol = symbol self.quantity = quantity self.price = price class TradeManager: def __init__(self): self.trades = [] def add_trade(self, symbol, quantity, price): trade = Trade(symbol, quantity, price) self.trades.append(trade) def get_trades(self): return self.trades def calculate_portfolio_value(self): total_value = 0 for trade in self.trades: total_value += trade.quantity * trade.price return total_value # 示例用法 trade_manager = TradeManager() trade_manager.add_trade("AAPL", 10, 150.0) trade_manager.add_trade("GOOG", 5, 2000.0) trades = trade_manager.get_trades() for trade in trades: print(f"Symbol: {trade.symbol}, Quantity: {trade.quantity}, Price: {trade.price}") portfolio_value = trade_manager.calculate_portfolio_value() print(f"Portfolio Value: {portfolio_value}")
时间: 2024-04-17 16:23:20 浏览: 26
这段代码是一个简单的交易管理系统的示例。它定义了两个类:`Trade`和`TradeManager`。
`Trade`类表示一个交易,具有三个属性:`symbol`代表交易的股票代码,`quantity`代表交易的数量,`price`代表交易的价格。
`TradeManager`类表示一个交易管理器,具有以下方法:
- `__init__`:初始化方法,创建一个空的交易列表。
- `add_trade`:添加一笔交易到交易列表中,通过传入股票代码、数量和价格来创建一个新的`Trade`对象,并将其添加到列表中。
- `get_trades`:返回交易列表。
- `calculate_portfolio_value`:计算并返回整个投资组合的价值,即所有交易的数量乘以价格的总和。
示例用法部分创建了一个`TradeManager`对象,并使用`add_trade`方法添加了两笔交易。然后,通过调用`get_trades`方法获取所有交易,并打印每笔交易的股票代码、数量和价格。最后,调用`calculate_portfolio_value`方法计算并打印整个投资组合的价值。
至于代码是否能正常运行,我无法在这个环境中直接运行代码,但根据代码逻辑来看,它应该能正常运行。
相关问题
用vnpy写一个通道分析方法的策略 你刚刚写到 if self.fast_k > self.slow
如果您想编写一个使用 VnPy 库的通道分析策略,您可以这样做:
```
from vnpy.trader.object import (TickData, BarData, TradeData, OrderData,
ContractData, PositionData)
from vnpy.trader.constant import Direction, Offset, OrderType
from vnpy.trader.engine import BaseStrategy
class ChannelAnalysisStrategy(BaseStrategy):
""""""
def __init__(self, engine, setting):
""""""
super().__init__(engine, setting)
self.fast_window = 10
self.slow_window = 20
self.fast_k = 0
self.slow_k = 0
self.long_entry = 0
self.short_entry = 0
self.long_exit = 0
self.short_exit = 0
def on_init(self):
"""
Callback when strategy is inited.
"""
pass
def on_start(self):
"""
Callback when strategy is started.
"""
self.write_log("策略启动")
def on_stop(self):
"""
Callback when strategy is stopped.
"""
self.write_log("策略停止")
def on_tick(self, tick: TickData):
"""
Callback of new tick data update.
"""
pass
def on_bar(self, bar: BarData):
"""
Callback of new bar data update.
"""
self.fast_k = self.fast_k * (self.fast_window - 1) / self.fast_window + bar.close * 2 / self.fast_window
self.slow_k = self.slow_k * (self.slow_window - 1) / self.slow_window + bar.close * 2 / self.slow_window
if self.fast_k > self.slow_k:
if self.pos == 0:
self.buy(bar.close, 1)
elif self.fast_k < self.slow_k:
if self.pos == 1:
self.sell(bar.close, 1)
def on_trade(self, trade: TradeData):
"""
Callback of new trade data update.
"""
pass
def on_order(self, order: OrderData):
"""
Callback of new order data update.
"""
pass
```
这是一个简单的通道分析策略的框架,您可以在其中添加更多的策略逻辑和判断条件。
现在有这样一个类 class PulicTopic: #成交记录 trade ={"method": "subscribe", "params": ["trade@btc_usdt"]} python在实例化PublicTopic时,怎么改变params里面的值
你可以在实例化 `PublicTopic` 的时候传入新的参数来改变 `params` 中的值。例如,假设你想要订阅 `depth@btc_usdt`,你可以这样做:
```python
class PublicTopic:
def __init__(self, params):
self.trade = {"method": "subscribe", "params": params}
# 实例化 PublicTopic,并订阅 depth@btc_usdt
public_topic = PublicTopic(["depth@btc_usdt"])
```
这样就会将 `params` 中的值改为 `["depth@btc_usdt"]`。
相关推荐
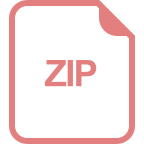
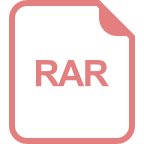












