输入一个正整数序列,遇到负数时停止,建立一个线性链表存储读入的数据,然后从键盘读入一数据x,在该链表中查找有无比x大的数据,有则全部输出,没有则输出“no found”
时间: 2024-10-01 08:08:26 浏览: 55
这个任务涉及链表操作和查找功能。首先,你需要创建一个单链表数据结构,用于存储输入的正整数序列。当遇到负数时,就不再添加到链表中。接着,用户会输入一个值 x,你需要遍历链表,查找是否存在比 x 更大的元素。
1. 初始化一个空的链表头节点(通常设为 `null`),并定义一个 `ListNode` 类表示链表的节点,包含一个数值 `value` 和指向下一个节点的引用 `next`。
```python
class ListNode:
def __init__(self, value=0, next=None):
self.value = value
self.next = next
```
2. 遍历输入流,将正整数加入链表,如果遇到负数,停止处理输入:
```python
def build_linked_list():
head = ListNode()
current = head
while True:
num = int(input("请输入一个正整数(输入负数结束):"))
if num < 0:
break
current.next = ListNode(num)
current = current.next
return head
```
3. 定义一个函数来搜索链表,并打印出所有大于给定值 x 的元素(如果有):
```python
def search_and_output(head, x):
current = head
while current is not None:
if current.value > x:
print(current.value, end=" ")
current = current.next
if current is None:
print("no found")
```
4. 主程序入口:
```python
if __name__ == "__main__":
head = build_linked_list()
x = int(input("请输入查找值 x:"))
search_and_output(head, x)
```
阅读全文
相关推荐
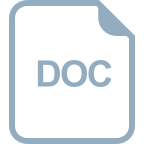
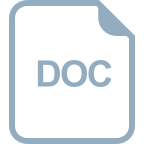
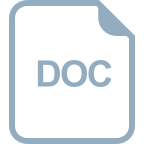




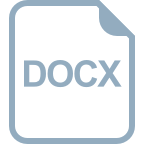
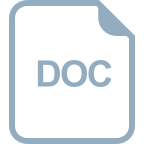
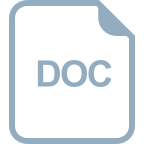
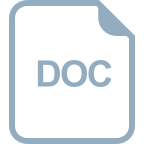
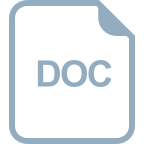
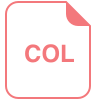



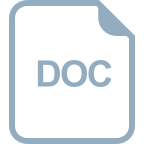
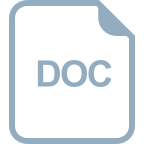
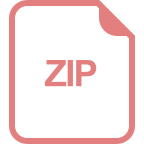