java解析excel中有三张表的数据
时间: 2024-04-14 20:29:18 浏览: 11
在Java中解析Excel文件可以使用Apache POI库。如果Excel文件中有多个表格,可以通过POI的Sheet对象来访问每个表格的数据。
下面是一个简单的示例代码,演示如何解析一个包含三个表格的Excel文件:
```java
import org.apache.poi.ss.usermodel.*;
import java.io.FileInputStream;
import java.io.IOException;
public class ExcelParser {
public static void main(String[] args) {
try {
// 读取Excel文件
FileInputStream file = new FileInputStream("path/to/excel.xlsx");
Workbook workbook = WorkbookFactory.create(file);
// 遍历每个表格
for (int i = 0; i < workbook.getNumberOfSheets(); i++) {
Sheet sheet = workbook.getSheetAt(i);
System.out.println("表格名称: " + sheet.getSheetName());
// 遍历每行数据
for (Row row : sheet) {
// 遍历每个单元格
for (Cell cell : row) {
// 获取单元格的值
CellType cellType = cell.getCellType();
if (cellType == CellType.STRING) {
System.out.print(cell.getStringCellValue() + "\t");
} else if (cellType == CellType.NUMERIC) {
System.out.print(cell.getNumericCellValue() + "\t");
} else if (cellType == CellType.BOOLEAN) {
System.out.print(cell.getBooleanCellValue() + "\t");
}
}
System.out.println();
}
System.out.println();
}
// 关闭文件流
workbook.close();
file.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请将代码中的 `"path/to/excel.xlsx"` 替换为你实际的Excel文件路径。这段代码会遍历每个表格,并打印出每个单元格的值。
注意:在运行代码之前,请确保已经将 Apache POI 的相关库文件添加到你的项目中。你可以通过 Maven 或手动下载 jar 文件来导入这些库。
相关推荐
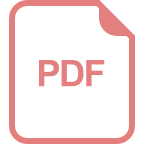














