写一个完整代码,使用using namespace std,编写一个二维数组类double A[m][n],通过函数计算该数组的最大值、最小值、均值,一并返回并打印输出,使用using namespace std的形式
时间: 2024-12-19 19:21:07 浏览: 14
```cpp
#include <iostream>
#include <vector>
class Array2D {
private:
int m;
int n;
std::vector<std::vector<double>> data;
public:
Array2D(int rows, int cols) : m(rows), n(cols) {
data.resize(m, std::vector<double>(n));
}
void setValue(int row, int col, double value) {
if (row >= 0 && row < m && col >= 0 && col < n) {
data[row][col] = value;
} else {
std::cout << "Invalid index!" << std::endl;
}
}
void printArray() const {
for (const auto &row : data) {
for (const double elem : row) {
std::cout << elem << " ";
}
std::cout << "\n";
}
}
double getMaxValue() const {
if (m == 0 || n == 0) return 0;
double max_val = data[0][0];
for (int i = 0; i < m; ++i) {
for (int j = 0; j < n; ++j) {
max_val = std::max(max_val, data[i][j]);
}
}
return max_val;
}
double getMinValue() const {
if (m == 0 || n == 0) return 0;
double min_val = data[0][0];
for (int i = 0; i < m; ++i) {
for (int j = 0; j < n; ++j) {
min_val = std::min(min_val, data[i][j]);
}
}
return min_val;
}
double getAverage() const {
if (m == 0 || n == 0) return 0;
double sum = 0;
for (int i = 0; i < m; ++i) {
for (int j = 0; j < n; ++j) {
sum += data[i][j];
}
}
return sum / (m * n);
}
void computeAndPrintMinMaxAvg() const {
double max_value = getMaxValue();
double min_value = getMinValue();
double average = getAverage();
std::cout << "Max Value: " << max_value << ", Min Value: " << min_value << ", Average: " << average << std::endl;
// If you want to print the array too, call this line after computing min and max
// printArray();
}
};
int main() {
int rows = 3, cols = 4;
Array2D arr(rows, cols);
// Set some values
arr.setValue(0, 0, 5.5);
arr.setValue(1, 2, 2.7);
arr.setValue(2, 3, 9.8);
arr.computeAndPrintMinMaxAvg();
return 0;
}
```
这段代码定义了一个名为`Array2D`的二维数组类,包含了初始化、设置元素值、打印数组、获取最大值、最小值和平均值的功能。`computeAndPrintMinMaxAvg`函数将这三项结果一起输出到控制台。
阅读全文
相关推荐
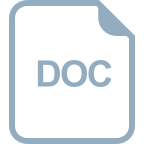
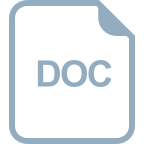
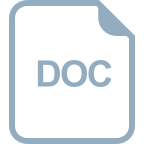















