两个LED灯交替闪烁代码c语言
时间: 2024-12-21 16:27:13 浏览: 0
在C语言中,要让两个LED灯交替闪烁,我们需要模拟这两个LED灯的状态并控制它们的开关。由于实际硬件操作通常通过IO端口完成,这里我们将假设我们正在处理一些模拟的LED灯状态。以下是一个简单的示例代码,它使用了定时器来进行交替闪烁:
```c
#include <stdio.h>
#include <stdlib.h>
#include <delay.h> // 假设这里有delay函数用于延时
#define LED_1 PORTB.0
#define LED_2 PORTB.1
// 定义LED灯状态枚举
enum LedState {
OFF = 0,
ON
};
void toggle_led(int led) {
if (led == LED_1) {
PORTB ^= (1 << LED_1);
} else {
PORTB ^= (1 << LED_2);
}
}
int main() {
enum LedState current_led = LED_1; // 初始点亮LED1
while (1) {
toggle_led(current_led); // 切换当前点亮的LED
delay_ms(500); // 等待一段时间
if (current_led == LED_1) { // 如果之前是LED1,切换到LED2
current_led = LED_2;
} else { // 如果之前是LED2,切换回LED1
current_led = LED_1;
}
}
return 0;
}
```
在这个例子中,`PORTB`是一个假设的I/O端口寄存器,`.0`和`.1`代表对应的LED引脚。`toggle_led()`函数会切换指定LED的状态,而主循环则会反复将LED灯状态切换两次,形成交替闪烁。
注意这只是一个简化的模拟,并未直接连接到物理LED,实际应用中需要配合合适的硬件平台和IO库来实现。
阅读全文
相关推荐
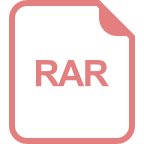
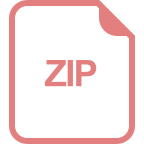
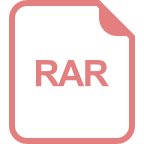

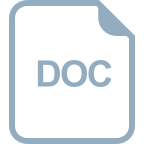
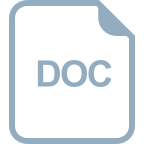












