private List<String> historyList = new ArrayList<>();设置该list数组只存5项,怎么存
时间: 2024-12-24 15:40:42 浏览: 7
如果你想要限制`ArrayList` `historyList`最多只能存储5项元素,可以在添加新元素时检查其长度。当列表已满5个元素时,不再添加新的元素。以下是使用Java的一个示例:
```java
private int maxHistorySize = 5;
private List<String> historyList = new ArrayList<>(maxHistorySize); // 初始化时指定最大容量
public void addItem(String item) {
if (historyList.size() < maxHistorySize) { // 如果未达到最大容量
historyList.add(item);
} else if (historyList.size() == maxHistorySize && !historyList.contains(item)) { // 若已满,且新元素不在列表中
historyList.remove(historyList.size() - 1); // 移除最后一个元素以腾出空间
historyList.add(0, item); // 将新元素放在最前面
}
}
```
在这个例子中,如果尝试添加第6个及以上元素,会自动替换最近的一项历史记录。
相关问题
public class DocumentService extends ServiceImpl<DocumentMapper, Document> { @Resource private DocumentMapper documentMapper; @Resource private CateMapper cateMapper; @Resource private HistoryMapper historyMapper; public List<Document> listDocument(PageInfo<?> pageInfo, Document document) { PageHelper.startPage(pageInfo.getCurrent(), pageInfo.getPageSize()); List<Document> documentList = documentMapper.selectList(Wrappers.<Document>lambdaQuery() .like(StringUtils.isNotBlank(document.getTitle()), Document::getTitle, document.getTitle()) .like(StringUtils.isNotBlank(document.getSummary()), Document::getSummary, document.getSummary()) .eq(document.getCateId() != null, Document::getCateId, document.getCateId()) .eq(document.getDeptId() != null, Document::getDeptId, document.getDeptId()) .eq(document.getActive() != null, Document::getActive, document.getActive()) .eq(document.getRecommend() != null, Document::getRecommend, document.getRecommend()) .eq(document.getCarousel() != null, Document::getCarousel, document.getCarousel()) .orderByDesc(Document::getDateTime) ); if (!documentList.isEmpty()) { List<Long> documentIdList = documentList.stream().map(Document::getCateId).collect(Collectors.toList()); Map<Long, Cate> cateMap = cateMapper.selectBatchIds(documentIdList).stream().collect(Collectors.toMap(Cate::getId, e -> e, (e1, e2) -> e2)); for (Document documentItem : documentList) { documentItem.setCate(cateMap.get(documentItem.getCateId())); } } return documentList; } public Document getDocumentById(Long id, HttpServletRequest request) { Document document = documentMapper.selectById(id); if (document == null) { return null; } document.setHits(document.getHits() + 1); documentMapper.updateById(document); document.setCate(cateMapper.selectById(document.getCateId())); History history = new History(); history.setDocumentId(id); history.setDateTime(LocalDateTime.now()); history.setUserId(Long.valueOf((String) StpUtil.getLoginId())); history.setIp(request.getRemoteAddr()); historyMapper.insert(history); return document; } public List<Document> listRecommend(Long id) { //Todo 推荐 return new ArrayList<>(); } public Boolean copyDocument(Long id) { Document document = documentMapper.selectById(id); if (document == null) { return false; } document.setDateTime(LocalDateTime.now()); document.setId(null); document.setDeptId(StpUtil.getSession().getModel(SaSession.USER, User.class).getBelongDeptId()); document.setHits(0L); document.setActive(false); document.setRecommend(false); int result = documentMapper.insert(document); return result == 1; } }
这是一个Java类的示例代码,名为`DocumentService`,它是一个服务类,提供了一些操作文档的方法。该类使用了Spring框架的一些注解,如`@Resource`和`@Autowired`来进行依赖注入。
在该类中,有一些方法可以执行不同的操作:
- `listDocument`方法用于获取文档列表,根据传入的条件进行筛选和排序。
- `getDocumentById`方法用于根据文档ID获取具体的文档信息,并更新文档的点击次数和记录历史信息。
- `listRecommend`方法用于获取推荐的文档列表(目前该方法返回一个空列表,还未实现具体逻辑)。
- `copyDocument`方法用于复制指定ID的文档,并创建一个新的副本。
需要注意的是,这段代码只是一个示例,并不能直接运行。你可以根据自己的业务需求和数据库结构来修改和完善这些方法。同时,还需要确保相关的Mapper类和实体类已经正确配置和引入。
可以用java语言帮我实现以下功能要求吗,要求如下:功能要求:编写一个类似ubuntu terminal的程序 详细要求: 1.有配置文件 miniShell.conf promote=long # 显示提示符 loginname@hostname$ or # or promote=short # 显示提示符 $ or # background=black font=宋体 fontsize=30 fontcolor=white 用户可以修改配置文件,设置字体、字号、颜色等。 2.支持常用命令: ls ls -l history pwd 3.可以向上翻动命令历史。 请给出完整java代码。
以下是一个基于你的要求编写的 Java 代码实现一个类似 Ubuntu 终端的程序:
```java
import java.io.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
import java.util.Scanner;
public class MiniShell {
private static String PROMPT;
private static String BACKGROUND;
private static String FONT;
private static int FONTSIZE;
private static String FONTCOLOR;
private static List<String> commandHistory;
public static void main(String[] args) {
loadConfig();
commandHistory = new ArrayList<>();
while (true) {
System.out.print(PROMPT);
String command = getInput();
if (command.equals("exit")) {
break;
}
if (command.equals("history")) {
printCommandHistory();
continue;
}
executeCommand(command);
}
}
private static void loadConfig() {
Properties properties = new Properties();
try {
properties.load(new FileInputStream("miniShell.conf"));
PROMPT = properties.getProperty("promote").equals("long") ? getLongPrompt() : getShortPrompt();
BACKGROUND = properties.getProperty("background");
FONT = properties.getProperty("font");
FONTSIZE = Integer.parseInt(properties.getProperty("fontsize"));
FONTCOLOR = properties.getProperty("fontcolor");
} catch (IOException e) {
e.printStackTrace();
}
}
private static String getLongPrompt() {
String loginName = System.getProperty("user.name");
String hostName = "";
try {
hostName = java.net.InetAddress.getLocalHost().getHostName();
} catch (java.net.UnknownHostException e) {
e.printStackTrace();
}
return loginName + "@" + hostName + "$ ";
}
private static String getShortPrompt() {
return "$ ";
}
private static String getInput() {
Scanner scanner = new Scanner(System.in);
String input = scanner.nextLine();
commandHistory.add(input);
return input;
}
private static void executeCommand(String command) {
try {
ProcessBuilder processBuilder = new ProcessBuilder(command.split(" "));
Process process = processBuilder.start();
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
process.waitFor();
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
private static void printCommandHistory() {
for (String command : commandHistory) {
System.out.println(command);
}
}
}
```
请确保将配置文件 `miniShell.conf` 放在与 Java 文件相同的目录下,并按照你的要求设置配置项。这个程序支持在终端输入命令,并执行常见的命令(如 `ls`、`ls -l`、`pwd`),并且可以显示命令历史记录。你可以根据需要自定义配置文件中的属性,来修改提示符的显示方式和其他外观设置。
阅读全文
相关推荐














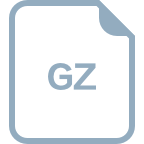