根据字符使用权值不同,设计哈夫曼编码,具体功能如下:①输入N个权值。②先构造哈夫曼树,然后再求各结点的编码。将编码写入文件③显示指定字符的哈夫曼编码④求指定两个结点的公共编码(先找到共同的祖先)。用C语言写出代码
时间: 2024-12-19 12:28:46 浏览: 15
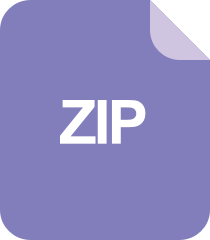
哈夫曼编码及解码(左子树权值小于右子树)

在C语言中,我们可以使用优先队列(通常通过二叉堆实现)来构建哈夫曼树并生成编码。以下是基本的步骤和部分代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <limits.h>
// 结构体表示节点,包含权值、左孩子和右孩子
typedef struct Node {
int weight;
struct Node *left, *right;
} Node;
// 创建新节点
Node* newNode(int weight) {
Node* node = (Node*)malloc(sizeof(Node));
node->weight = weight;
node->left = node->right = NULL;
return node;
}
// 比较节点权值大小
int compare(const void* a, const void* b) {
return ((const Node*)a)->weight - ((const Node*)b)->weight;
}
// 构建最小堆
void heapify(Node** root, int size, int i) {
Node* smallest = root[i];
int l = 2 * i + 1; // 左孩子
int r = 2 * i + 2; // 右孩子
if (l < size && smallest->weight > root[l]->weight)
smallest = root[l];
if (r < size && smallest->weight > root[r]->weight)
smallest = root[r];
if (smallest != root[i]) {
swap(root[i], smallest);
heapify(root, size, root[i]->weight == smallest->weight ? l : r);
}
}
// 插入元素到堆
void insert(Node** root, int weight) {
Node* newNode = newNode(weight);
Node* temp = *root;
(*root) = newNode;
heapify(root, 1, 0);
while (temp && temp->weight < newNode->weight) {
Node* parent = temp;
temp = temp->weight < newNode->weight ? temp->left : temp->right;
if (parent->weight == newNode->weight)
break;
swap(parent, temp);
}
}
// 获取哈夫曼树根
Node* buildHuffmanTree(int arr[], int n) {
for (int i = 0; i < n; ++i)
insert(&heap, arr[i]);
return heap[0];
}
// 计算节点编码
void calculateCode(Node* root, char* code, int prefix) {
if (!root)
return;
if (root->left == NULL && root->right == NULL) {
code[root->weight] = (char)(prefix + '0');
printf("字符 %d 的编码是:%c\n", root->weight, code[root->weight]);
} else {
calculateCode(root->left, code, prefix * 2);
calculateCode(root->right, code, prefix * 2 + 1);
}
}
// 主函数入口
int main() {
int N;
printf("请输入字符的数量(即权值的数量): ");
scanf("%d", &N);
int weights[N]; // 权值数组
for (int i = 0; i < N; i++) {
printf("请输入第%d个字符的权值: ", i+1);
scanf("%d", &weights[i]);
}
Node* huffmanRoot = buildHuffmanTree(weights, N); // 构建哈夫曼树
char code[MAX权值 + 1]; // 初始化编码数组
calculateCode(huffmanRoot, code, 0); // 计算编码并打印
// 其他功能如保存编码到文件,查找特定字符编码等可以添加相应代码实现,这里不再详述。
return 0;
}
```
这个代码片段实现了构建哈夫曼树以及计算节点编码的基本过程,你可以根据需要扩展其他功能。注意,`MAX权值 + 1` 应替换为你预计的最大权值,并确保它足够大以容纳所有权重。另外,对于保存编码到文件和查找特定字符编码的功能,你还需要实现相应的I/O操作和辅助函数。
阅读全文
相关推荐
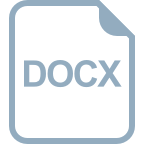
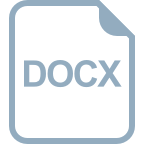

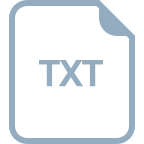
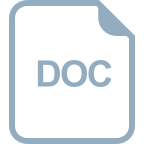
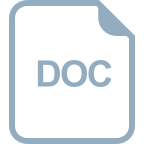
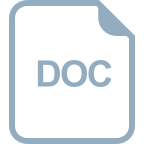
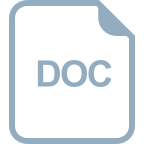









