字符串提取ip地址 用c语言
时间: 2024-09-19 21:15:54 浏览: 66
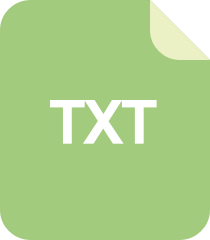
linux获取IP.txt
在C语言中,提取字符串中的IP地址通常涉及到正则表达式或者遍历字符串查找特定模式。这里是一个简单的示例,展示如何使用`strtok()`函数配合正则表达式来实现这个功能:
```c
#include <stdio.h>
#include <string.h>
#include <regex.h>
// 定义一个函数来检查并提取IP地址
void extract_ip(const char *str) {
regex_t ip_regex;
char token[INET_ADDRSTRLEN];
char *token_ptr;
// 创建正则表达式匹配IPv4地址
if (regcomp(&ip_regex, "^([0-9]{1,3}\\.){3}[0-9]{1,3}$", REG_EXTENDED | REG_ICASE) != 0) {
fprintf(stderr, "Error compiling regex\n");
return;
}
// 分割字符串,寻找符合IP地址的片段
token_ptr = strtok(str, ".");
while (token_ptr != NULL) {
// 检查是否匹配IP地址格式
if (regexec(&ip_regex, token_ptr, 0, NULL, 0) == 0) {
inet_pton(AF_INET, token_ptr, &token); // 转换为网络字节序
printf("Found IP address: %s\n", inet_ntop(AF_INET, &token, token, INET_ADDRSTRLEN));
}
token_ptr = strtok(NULL, ".");
}
// 清理正则表达式
regfree(&ip_regex);
}
int main() {
const char *input_str = "Here is an IP address: 192.168.1.1 and another one: 255.255.255.255";
extract_ip(input_str);
return 0;
}
```
这个例子假设输入字符串中的IP地址是由点分十进制形式组成的,并且每个部分都在0到255之间。如果需要处理其他格式或有特殊需求,可能需要调整正则表达式。
阅读全文
相关推荐
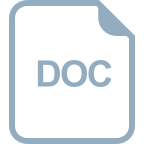
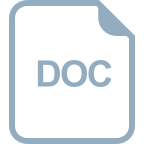
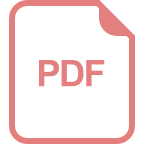
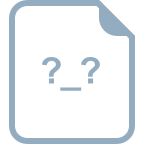
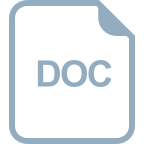
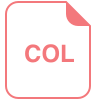







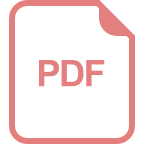
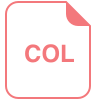

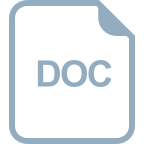