c++代码示例:设平面上有n个点P1,P2,...,Pn,n>1,Pi的直角坐标是(xi,yi),i=1,2,...n,求距离最近的两个点以及它们之间的距离
时间: 2024-10-11 17:07:48 浏览: 33
在C++中,可以使用一个简单的遍历和比较的方式来找到最接近的两个点。这里我们可以创建一个结构体Point来存储每个点的坐标,并实现一个函数findNearestPair()来解决问题。以下是示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
// 定义点的结构体
struct Point {
int x;
int y;
double distance_to(const Point &other) const {
return std::sqrt(std::pow(other.x - x, 2) + std::pow(other.y - y, 2));
}
};
// 找出最接近的两个点及其距离
std::pair<Point, double> findNearestPair(const std::vector<Point>& points) {
if (points.size() <= 1) {
throw std::runtime_error("At least two points are required");
}
Point min_distance_point = points[0];
double min_distance = points[0].distance_to(points[1]);
for (size_t i = 1; i < points.size(); ++i) {
double new_distance = points[i].distance_to(min_distance_point);
if (new_distance < min_distance) {
min_distance = new_distance;
min_distance_point = points[i];
}
}
return {min_distance_point, min_distance};
}
int main() {
std::vector<Point> points = {{1, 2}, {3, 4}, {5, 6}, {7, 8}}; // 假设这是给定的点集合
auto nearest_pair = findNearestPair(points);
std::cout << "The nearest pair is (" << nearest_pair.first.x << ", " << nearest_pair.first.y << ") and the distance is " << nearest_pair.second << std::endl;
return 0;
}
```
这个程序首先初始化最近的距离和点为第一个点,然后遍历所有其他点,如果发现新的点对之间的距离更小,就更新这两个值。最后返回最接近的那一对点及其距离。
阅读全文
相关推荐
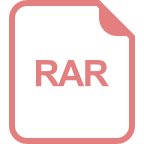
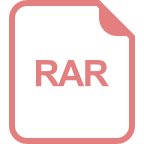
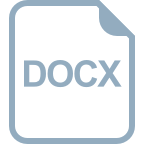
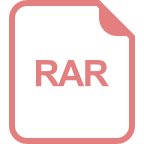
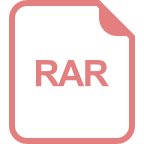
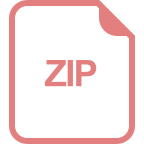
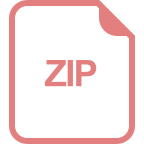
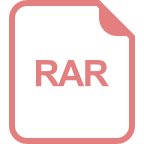
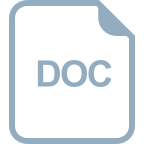
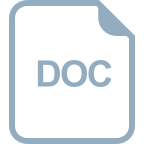
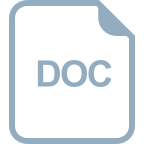
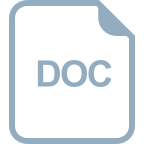
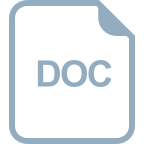
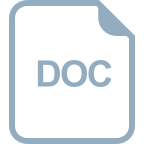
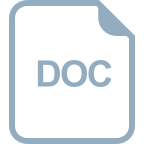
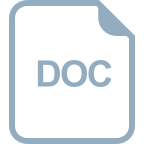
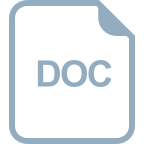
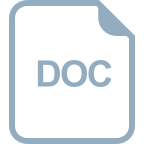
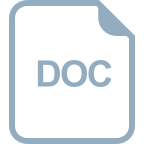