如何使用smtplib模块发送电子邮件及附件?
时间: 2024-05-02 17:18:08 浏览: 119
1. 导入smtplib模块和MIME模块
```python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
```
2. 设置发件人、收件人、主题和正文
```python
sender = '发件人邮箱地址'
receiver = '收件人邮箱地址'
subject = '邮件主题'
body = '邮件正文'
```
3. 创建MIMEMultipart对象并设置发件人、收件人和主题
```python
message = MIMEMultipart()
message['From'] = sender
message['To'] = receiver
message['Subject'] = subject
```
4. 创建MIMEText对象并设置正文
```python
text = MIMEText(body)
message.attach(text)
```
5. 创建MIMEApplication对象并设置附件
```python
with open('附件文件路径', 'rb') as f:
attachment = MIMEApplication(f.read(), _subtype='pdf')
attachment.add_header('Content-Disposition', 'attachment', filename='附件文件名.pdf')
message.attach(attachment)
```
6. 连接SMTP服务器并登录,发送邮件
```python
smtp_server = 'SMTP服务器地址'
smtp_port = 25 # SMTP服务器端口号
smtp_user = 'SMTP服务器用户名'
smtp_password = 'SMTP服务器密码'
smtp = smtplib.SMTP(smtp_server, smtp_port)
smtp.login(smtp_user, smtp_password)
smtp.sendmail(sender, receiver, message.as_string())
smtp.quit()
```
完整代码示例:
```python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
sender = '发件人邮箱地址'
receiver = '收件人邮箱地址'
subject = '邮件主题'
body = '邮件正文'
message = MIMEMultipart()
message['From'] = sender
message['To'] = receiver
message['Subject'] = subject
text = MIMEText(body)
message.attach(text)
with open('附件文件路径', 'rb') as f:
attachment = MIMEApplication(f.read(), _subtype='pdf')
attachment.add_header('Content-Disposition', 'attachment', filename='附件文件名.pdf')
message.attach(attachment)
smtp_server = 'SMTP服务器地址'
smtp_port = 25 # SMTP服务器端口号
smtp_user = 'SMTP服务器用户名'
smtp_password = 'SMTP服务器密码'
smtp = smtplib.SMTP(smtp_server, smtp_port)
smtp.login(smtp_user, smtp_password)
smtp.sendmail(sender, receiver, message.as_string())
smtp.quit()
```
阅读全文
相关推荐
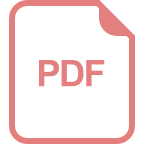
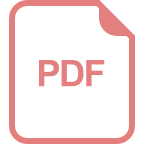
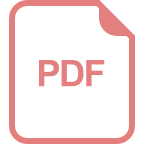
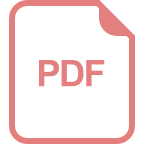
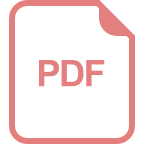
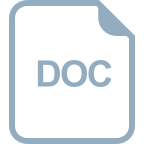
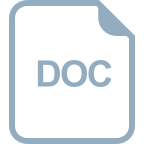
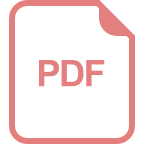
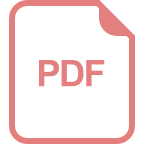
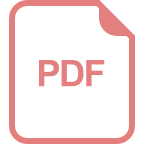
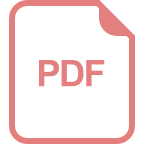
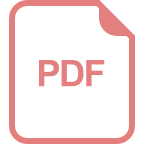
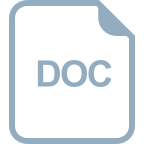
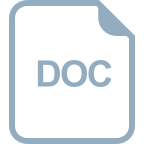
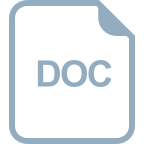
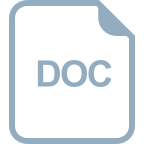
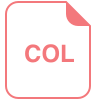