#include<iostream> #include<vector> using namespace std; class ChessGame { private: Man* man; AI* ai; Chess* chess; public: ChessGame(Man *man, AI *ai, Chess *chess); void play(); }; #include"Man.h" #include"AI.h" #include"Chess.h" #include "ChessGame.h" ChessGame::ChessGame(Man *man,AI *ai ,Chess *chess) { this->man = man; this->ai = ai; this->chess = chess; ai->init(chess); man->init(chess);//初始化棋手 } //对局开始五子棋游戏 void ChessGame::play() { Chess* chess=NULL; Man* man=NULL; AI* ai=NULL; chess->init(); while (1) { //先有棋手走棋 man->go(); if (chess->checkOver()) { chess->init(); continue; } //由Ai走 ai->go(); if (chess->checkOver()) { chess->init(); continue; } } }检查纠错
时间: 2024-02-21 10:58:52 浏览: 18
在代码中有几个问题:
1. 在 ChessGame 类中,Man、AI 和 Chess 类的头文件没有被包括进来,需要添加头文件。
2. 在 ChessGame 类中的 play() 函数中,chess、man 和 ai 没有被初始化,需要将它们初始化为构造函数中传递进来的变量。
3. 在 play() 函数中,当 checkOver() 函数返回 true 时,应该使用 break 语句跳出循环,而不是使用 continue 语句继续循环。
4. 在 play() 函数中,由于 chess、man 和 ai 变量已经在类的成员变量中定义,不需要再次定义。
下面是修改后的代码:
```
#include<iostream>
#include<vector>
#include"Man.h"
#include"AI.h"
#include"Chess.h"
using namespace std;
class ChessGame {
private:
Man* man;
AI* ai;
Chess* chess;
public:
ChessGame(Man* man, AI* ai, Chess* chess);
void play();
};
ChessGame::ChessGame(Man* man, AI* ai, Chess* chess) {
this->man = man;
this->ai = ai;
this->chess = chess;
ai->init(chess);
man->init(chess);
}
void ChessGame::play() {
chess->init();
while (1) {
//先有棋手走棋
man->go();
if (chess->checkOver()) {
chess->init();
break;
}
//由Ai走
ai->go();
if (chess->checkOver()) {
chess->init();
break;
}
}
}
int main() {
Man* man = new Man();
AI* ai = new AI();
Chess* chess = new Chess();
ChessGame game(man, ai, chess);
game.play();
delete man;
delete ai;
delete chess;
return 0;
}
```
相关推荐
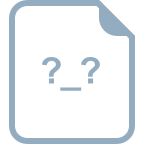















