用c语言,创建单项循环链表实现约瑟夫环,并分成头文件和源文件以及主函数
时间: 2024-05-08 10:16:42 浏览: 17
头文件:josephus.h
```c
#ifndef JOSEPHUS_H
#define JOSEPHUS_H
typedef struct node {
int number;
struct node *next;
} Node;
void createList(Node **head, int n);
void printList(Node *head);
void josephus(Node **head, int k);
#endif
```
源文件:josephus.c
```c
#include <stdio.h>
#include <stdlib.h>
#include "josephus.h"
void createList(Node **head, int n) {
Node *prev, *cur;
*head = (Node *)malloc(sizeof(Node));
(*head)->number = 1;
prev = *head;
for (int i = 2; i <= n; i++) {
cur = (Node *)malloc(sizeof(Node));
cur->number = i;
prev->next = cur;
prev = cur;
}
prev->next = *head;
}
void printList(Node *head) {
Node *cur = head;
do {
printf("%d ", cur->number);
cur = cur->next;
} while (cur != head);
printf("\n");
}
void josephus(Node **head, int k) {
Node *cur = *head, *prev;
while (cur->next != cur) {
for (int i = 1; i < k; i++) {
prev = cur;
cur = cur->next;
}
prev->next = cur->next;
printf("%d ", cur->number);
free(cur);
cur = prev->next;
}
*head = cur;
printf("%d\n", cur->number);
}
```
主函数:main.c
```c
#include <stdio.h>
#include "josephus.h"
int main() {
int n, k;
Node *head = NULL;
printf("Please input n and k: ");
scanf("%d%d", &n, &k);
createList(&head, n);
printf("The initial list is: ");
printList(head);
printf("The Josephus sequence is: ");
josephus(&head, k);
return 0;
}
```
相关推荐
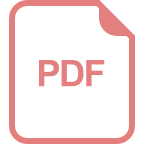
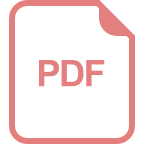
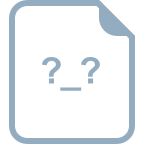














