类类型 /声明一个学生类类型: 数据成员:姓名(Char”)、年龄(short): 提供:构造函数、析构函数、拷贝构造函数; 功能:实现对一名学生信息的输入输出、实现对20名学生信息的输入输出。
时间: 2023-05-24 10:03:28 浏览: 121
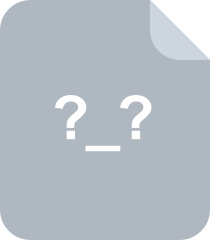
一个 Student类:包括姓名、学号、性别、专业、家庭地址。
A:
以下是一个简单的C++代码,用于声明一个学生类类型并实现各种功能。
```
#include <iostream>
using namespace std;
class Student {
public:
// 构造函数
Student(char* name, short age) {
this->name = new char[strlen(name) + 1];
strcpy(this->name, name);
this->age = age;
}
// 拷贝构造函数
Student(const Student& other) {
this->name = new char[strlen(other.name) + 1];
strcpy(this->name, other.name);
this->age = other.age;
}
// 析构函数
~Student() {
delete[] name;
}
// 输入输出信息
friend ostream& operator<<(ostream& out, const Student& student) {
out << "Name: " << student.name << endl;
out << "Age: " << student.age << endl;
return out;
}
friend istream& operator>>(istream& in, Student& student) {
cout << "Enter Name: ";
in >> student.name;
cout << "Enter Age: ";
in >> student.age;
return in;
}
private:
char* name;
short age;
};
int main() {
Student s1("Tom", 18);
cout << s1; // 输出学生信息
Student s2(s1); // 拷贝构造函数
cout << s2; // 输出学生信息
// 输入20个学生信息
Student students[20];
for (int i = 0; i < 20; i++) {
cout << "Enter Student " << i + 1 << " Information: " << endl;
cin >> students[i];
}
// 输出20个学生信息
for (int i = 0; i < 20; i++) {
cout << students[i] << endl;
}
return 0;
}
```
以上代码声明了一个名为`Student`的类类型,其中包括一个带有两个参数`name`和`age`的构造函数、一个拷贝构造函数和一个析构函数。此外,该类还含有输入输出信息的函数,以实现对一名学生信息的输入输出和对20名学生信息的输入输出。
阅读全文
相关推荐
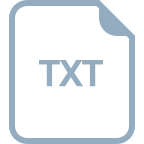
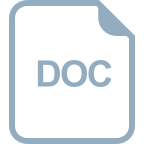
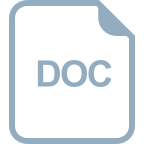
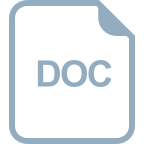
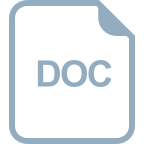
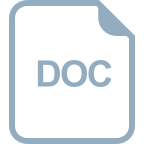
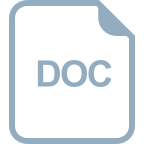
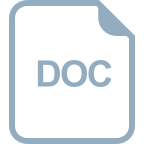
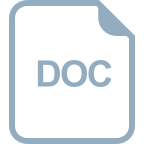
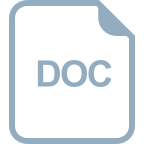
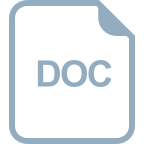
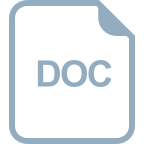
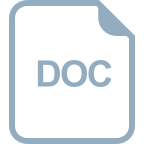
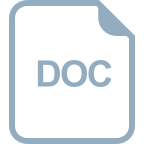
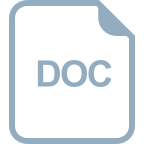
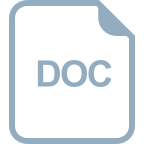
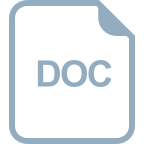
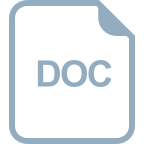