python 写个 RSA加密解密
时间: 2023-07-04 19:25:36 浏览: 56
好的,下面是一个简单的 Python RSA加密解密程序:
```python
import random
import math
# 模幂运算:计算 (base^exp) % mod
def exp_mode(base, exp, mod):
result = 1
while exp > 0:
if exp % 2 == 1:
result = (result * base) % mod
base = (base * base) % mod
exp = exp // 2
return result
# 判断是否为质数
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(math.sqrt(n)) + 1):
if n % i == 0:
return False
return True
# 生成指定位数的质数
def generate_prime(bit):
while True:
p = random.randint(2**(bit-1), 2**bit-1)
if is_prime(p):
return p
# 求最大公约数
def gcd(a, b):
if b == 0:
return a
else:
return gcd(b, a % b)
# 扩展欧几里得算法
def exgcd(a, b):
if b == 0:
return 1, 0, a
else:
x, y, gcd = exgcd(b, a % b)
return y, x - (a // b) * y, gcd
# 生成 RSA公钥和私钥
def generate_rsa_key(bit):
p = generate_prime(bit // 2)
q = generate_prime(bit // 2)
n = p * q
phi = (p - 1) * (q - 1)
e = 65537
d, _, _ = exgcd(e, phi)
d = (d % phi + phi) % phi
return (e, n), (d, n)
# RSA加密
def rsa_encrypt(plaintext, public_key):
e, n = public_key
ciphertext = [exp_mode(ord(c), e, n) for c in plaintext]
return ciphertext
# RSA解密
def rsa_decrypt(ciphertext, private_key):
d, n = private_key
plaintext = [chr(exp_mode(c, d, n)) for c in ciphertext]
return ''.join(plaintext)
# 测试代码
if __name__ == '__main__':
# 生成 RSA公钥和私钥
public_key, private_key = generate_rsa_key(1024)
# 显示公钥和私钥
print('Public key:', public_key)
print('Private key:', private_key)
# 明文和密文
plaintext = 'Hello, world!'
ciphertext = rsa_encrypt(plaintext, public_key)
decrypted_plaintext = rsa_decrypt(ciphertext, private_key)
# 显示加密和解密结果
print('Plaintext:', plaintext)
print('Ciphertext:', ciphertext)
print('Decrypted plaintext:', decrypted_plaintext)
```
上面的程序实现了基本的 RSA加密解密功能,生成了一个 1024位的 RSA公钥和私钥,对明文进行加密后再解密,最后输出加密前后的结果。
相关推荐
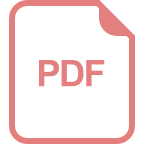
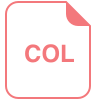
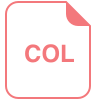
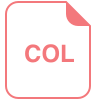
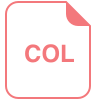
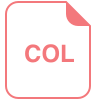






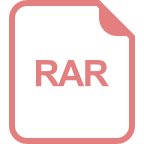
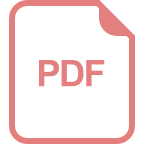
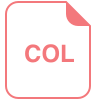