nsga2算法matlab代码实例
时间: 2023-09-05 12:13:48 浏览: 175
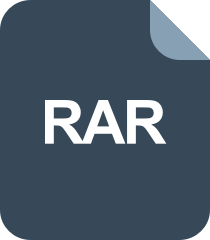
NSGA-2 matlab 实例
以下是NSGA-II算法的MATLAB代码示例:
```matlab
function [pop, front] = nsga2(pop, nObj, nPop, nGen, crossRate, mutRate, bounds)
% NSGA-II Algorithm
% pop: population, front: non-dominated fronts
% nObj: number of objectives
% nPop: population size
% nGen: number of generations
% crossRate: crossover rate
% mutRate: mutation rate
% bounds: decision variable bounds
% Initialization
for i = 1:nPop
pop(i).x = rand(1, nObj) .* (bounds(:,2)' - bounds(:,1)') + bounds(:,1)';
pop(i).f = evaluate(pop(i).x);
pop(i).rank = 0;
pop(i).crowding = 0;
end
% Evolution
for gen = 1:nGen
% Non-dominated sorting
[pop, front] = non_dominated_sorting(pop);
% Crowding distance assignment
pop = crowding_distance_assignment(pop, front);
% Offspring generation
for i = 1:2:nPop
% Select parents
p1 = tournament_selection(pop, front);
p2 = tournament_selection(pop, front);
% Crossover
if rand < crossRate
[c1, c2] = simulated_binary_crossover(p1.x, p2.x);
else
c1 = p1.x;
c2 = p2.x;
end
% Mutation
if rand < mutRate
c1 = polynomial_mutation(c1, bounds);
end
if rand < mutRate
c2 = polynomial_mutation(c2, bounds);
end
% Create offspring
pop(i+nPop).x = c1;
pop(i+nPop).f = evaluate(c1);
pop(i+nPop).rank = 0;
pop(i+nPop).crowding = 0;
pop(i+nPop+1).x = c2;
pop(i+nPop+1).f = evaluate(c2);
pop(i+nPop+1).rank = 0;
pop(i+nPop+1).crowding = 0;
end
% Merge parent and offspring populations
pop = [pop(1:nPop), pop(nPop+1:end)];
end
% Final non-dominated sorting
[pop, front] = non_dominated_sorting(pop);
end
function f = evaluate(x)
% Objective function
f(1) = x(1)^2 + x(2)^2;
f(2) = (x(1)-1)^2 + x(2)^2;
end
function [pop, front] = non_dominated_sorting(pop)
% Non-dominated sorting
nPop = length(pop);
% Initialize fronts and domination counts
front = {};
nDom = zeros(nPop, 1);
% Calculate domination counts
for i = 1:nPop
for j = 1:nPop
if i ~= j
if dominates(pop(i), pop(j))
nDom(i) = nDom(i) + 1;
end
end
end
end
% Assign individuals to first front
front{1} = find(nDom == 0);
% Assign individuals to other fronts
iFront = 1;
while ~isempty(front{iFront})
iFront = iFront + 1;
for i = 1:length(front{iFront-1})
p = front{iFront-1}(i);
for j = 1:nPop
if nDom(j) > 0
nDom(j) = nDom(j) - 1;
if nDom(j) == 0
front{iFront} = [front{iFront}, j];
end
end
end
end
end
% Assign ranks to population
for i = 1:length(front)
for j = 1:length(front{i})
pop(front{i}(j)).rank = i;
end
end
end
function pop = crowding_distance_assignment(pop, front)
% Crowding distance assignment
nPop = length(pop);
nObj = length(pop(1).f);
for i = 1:length(front)
nFront = length(front{i});
if nFront > 2
% Initialize crowding distance
for j = 1:nFront
pop(front{i}(j)).crowding = 0;
end
% Calculate crowding distance for each objective
for m = 1:nObj
% Sort individuals by objective value
[~, order] = sort([pop(front{i}).f(m)]);
% Assign infinite crowding distance to boundary individuals
pop(front{i}(order(1))).crowding = Inf;
pop(front{i}(order(end))).crowding = Inf;
% Calculate crowding distance for non-boundary individuals
for j = 2:nFront-1
pop(front{i}(order(j))).crowding = ...
pop(front{i}(order(j))).crowding + ...
(pop(front{i}(order(j+1))).f(m) - pop(front{i}(order(j-1))).f(m)) / ...
(pop(front{i}(order(end))).f(m) - pop(front{i}(order(1))).f(m));
end
end
end
end
end
function b = dominates(p1, p2)
% Dominance comparison
f1 = p1.f;
f2 = p2.f;
if all(f1 <= f2) && any(f1 < f2)
b = true;
elseif all(f2 <= f1) && any(f2 < f1)
b = false;
else
b = false;
end
end
function p = tournament_selection(pop, front)
% Tournament selection
nPop = length(pop);
nFronts = length(front);
% Select random individuals
i1 = randi(nPop);
i2 = randi(nPop);
% Ensure that individuals are in different fronts
while pop(i1).rank == pop(i2).rank
i2 = randi(nPop);
end
% Select individual with lower rank
if pop(i1).rank < pop(i2).rank
p = pop(i1);
else
p = pop(i2);
end
end
function [c1, c2] = simulated_binary_crossover(p1, p2)
% Simulated binary crossover
nVar = length(p1);
c1 = zeros(1, nVar);
c2 = zeros(1, nVar);
for i = 1:nVar
if rand < 0.5
if abs(p1(i) - p2(i)) > 1e-10
if p1(i) < p2(i)
y1 = p1(i);
y2 = p2(i);
else
y1 = p2(i);
y2 = p1(i);
end
beta = 1 + (2 * (y1 - bounds(i,1)) / (y2 - y1));
alpha = 2 - beta^(-(1+1));
u = rand;
if u <= (1 / alpha)
c1(i) = y1 + (y2 - y1) * (u * alpha)^(1/(1+1));
else
c1(i) = y1 + (y2 - y1) * (1/(2-u*alpha))^(1/(1+1));
end
u = rand;
if u <= (1 / alpha)
c2(i) = y1 + (y2 - y1) * (u * alpha)^(1/(1+1));
else
c2(i) = y1 + (y2 - y1) * (1/(2-u*alpha))^(1/(1+1));
end
else
c1(i) = p1(i);
c2(i) = p2(i);
end
else
c1(i) = p1(i);
c2(i) = p2(i);
end
end
end
function c = polynomial_mutation(p, bounds)
% Polynomial mutation
nVar = length(p);
c = p;
for i = 1:nVar
if rand < 1/nVar
u = rand;
if u <= 0.5
delta = (2*u)^(1/(1+20)) - 1;
else
delta = 1 - (2*(1-u))^(1/(1+20));
end
c(i) = p(i) + delta * (bounds(i,2) - bounds(i,1));
end
end
end
```
这是一个简单的双目标NSGA-II示例代码,其中包括选择、交叉、变异、非支配排序和拥挤距离分配。要使用此代码,您需要编写自己的目标函数,该函数将决策变量作为输入并返回目标函数值。您还需要指定一些算法参数,例如种群大小,交叉率和变异率。最后,您可以运行算法并获得一组非支配解以及它们所在的前沿。
阅读全文
相关推荐
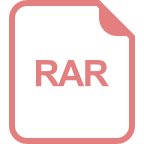
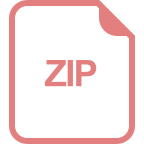
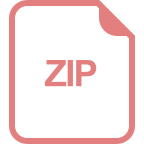

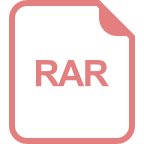
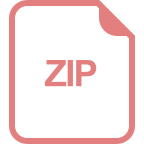
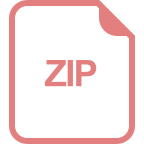
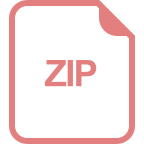
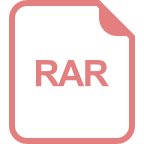
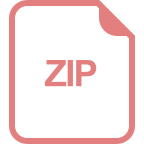
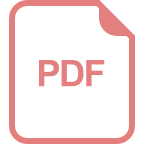
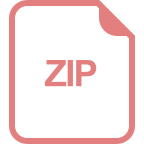
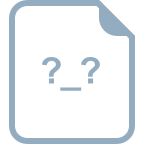
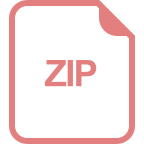
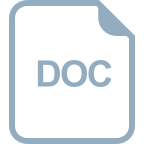
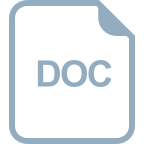
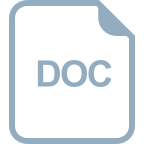
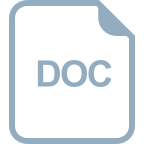