合并list中的numpy数组
时间: 2023-11-18 08:03:12 浏览: 37
要合并list中的numpy数组,可以使用numpy库中的concatenate函数。这个函数可以将多个数组沿着指定的轴进行连接。
下面是一个简单的示例代码:
```python
import numpy as np
# 创建两个numpy数组
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
# 将两个数组合并成一个列表
lst = [arr1, arr2]
# 沿着第一个轴连接数组
merged_arr = np.concatenate(lst, axis=0)
print(merged_arr)
```
输出结果:
```
[1 2 3 4 5 6]
```
在示例代码中,首先创建了两个numpy数组arr1和arr2。然后将这两个数组合并成一个列表lst。最后使用concatenate函数将lst中的数组沿着第一个轴进行连接,生成一个新的numpy数组merged_arr。
相关问题
转化numpy数组
要将Python中的列表或其他数据结构转换为NumPy数组,需要使用NumPy库的array方法。以下是一个示例代码,将一个Python列表转换为NumPy数组:
```python
import numpy as np
my_list = [1, 2, 3, 4, 5]
my_array = np.array(my_list)
print(my_array)
```
输出:
```
[1 2 3 4 5]
```
您也可以将多个列表合并为一个多维数组:
```python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
my_array = np.array([list1, list2, list3])
print(my_array)
```
输出:
```
[[1 2 3]
[4 5 6]
[7 8 9]]
```
请注意,NumPy数组中的所有元素必须具有相同的类型。如果列表包含不同类型的元素,则NumPy将尝试将它们全部转换为同一类型。
如果我的数组不是numpy数组,只是普通数组呢
如果你的数组不是`numpy`数组,而是普通的Python列表,你可以使用`extend`方法来在一个维度上合并它们。以下是一个示例代码:
```python
# 创建两个普通的列表
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# 在第一个维度上合并列表
list1.extend(list2)
print(list1)
```
输出结果为:
```
[1, 2, 3, 4, 5, 6]
```
在这个例子中,我们使用`extend`方法将`list2`合并到`list1`中,实现了在第一个维度上合并列表的效果。
相关推荐
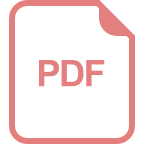
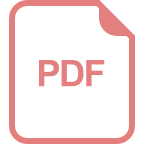













