用java使用对象graphics在坐标点(x1,y1)和(x2,y2)这两点之间绘制一条直线的实现代码为
时间: 2023-05-31 11:07:59 浏览: 144
以下是用Java绘制一条直线的实现代码:
``` java
import java.awt.Graphics;
public class DrawLine {
public static void main(String[] args) {
int x1 = 10, y1 = 10, x2 = 100, y2 = 100;
DrawingPanel panel = new DrawingPanel(200, 200);
Graphics g = panel.getGraphics();
g.drawLine(x1, y1, x2, y2);
}
}
```
在这个例子中,我们创建了一个名为 `DrawLine` 的类,在 `main` 方法中定义了需要绘制直线的起点和终点坐标。
我们使用 `DrawingPanel` 类来创建一个绘图面板,并使用 `getGraphics` 方法获取绘图上下文对象。然后,我们使用 `drawLine` 方法在指定的起点和终点之间绘制一条直线。
需要注意的是,在使用 `DrawingPanel` 类的时候,需要下载相应的jar包,并将其添加到项目的classpath中。
相关问题
import java.awt.Color;import java.awt.Graphics;import javax.swing.JFrame;import javax.swing.JPanel;public class BresenhamAlgorithm extends JPanel { private static final long serialVersionUID = 1L; public void paint(Graphics g) { // 画第一条线 drawLine(g, 50, 150, 100, 50, Color.MAGENTA); // 画第二条线 drawLine(g, 150, 150, 100, 50, Color.PINK); // 画第三条线 drawLine(g, 75, 100, 125, 100, Color.RED); } private void drawLine(Graphics g, int x1, int y1, int x2, int y2, Color color) { int dx = Math.abs(x2 - x1); int dy = Math.abs(y2 - y1); int sx = x1 < x2 ? 1 : -1; int sy = y1 < y2 ? 1 : -1; int err = dx - dy; while (true) { g.setColor(color); g.drawLine(x1, y1, x1, y1); if (x1 == x2 && y1 == y2) { break; } int e2 = 2 * err; if (e2 > -dy) { err -= dy; x1 += sx; } if (e2 < dx) { err += dx; y1 += sy; } } } public static void main(String[] args) { JFrame frame = new JFrame("Bresenham Algorithm"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(300, 300); BresenhamAlgorithm panel = new BresenhamAlgorithm(); frame.add(panel); frame.setVisible(true); }}解释每一行代码
我可以回答这个问题。这是一个使用Bresenham算法绘制直线的Java程序。它在一个JFrame中创建了一个JPanel,并在其中绘制了三条线。Bresenham算法是一种用于计算计算机图形学中的直线的算法。
addMouseMotionListener(new MouseMotionListener() { public void mouseMoved(MouseEvent event){ }; public void mouseDragged(MouseEvent event){ Graphics2D g=(Graphics2D)getGraphics(); x2=event.getX(); y2=event.getY(); if(flag==1) { red=Integer.parseInt(jt1.getText()); green=Integer.parseInt(jt2.getText()); blue=Integer.parseInt(jt3.getText()); Color c=new Color(red,green,blue); g.setColor(c); g.draw(new Line2D.Double(x1,y1,x2,y2)); } x1=x2; y1=y2; label.setText("当前鼠标的位置:("+ x2 + "," + y2 +")"); g.dispose(); } });代码分析
这段代码添加了一个鼠标移动和拖拽事件监听器,其中的`mouseMoved`方法在鼠标移动时被触发,`mouseDragged`方法在鼠标拖拽时被触发。
在`mouseMoved`方法中,没有实现任何功能,只是空方法体。在`mouseDragged`方法中,首先获取了`Graphics2D`对象,然后获取鼠标的当前坐标`x2`和`y2`,接着判断`flag`标志,如果为1,则根据文本框中的RGB值设置画笔颜色,绘制一条直线,然后更新`x1`和`y1`的值为当前坐标,更新界面上的标签显示当前鼠标位置,最后释放`Graphics2D`对象。
需要注意的是,这段代码中的绘制操作并不是线程安全的,因此在实际开发中需要注意线程安全问题。
阅读全文
相关推荐
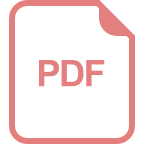
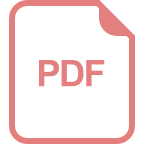
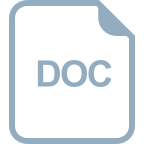
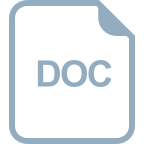
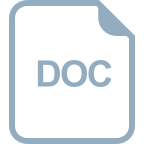
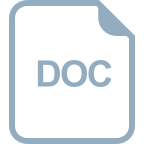
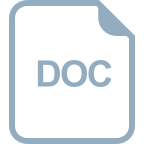






