addMouseMotionListener(new MouseMotionListener() { public void mouseMoved(MouseEvent event){ }; public void mouseDragged(MouseEvent event){ Graphics2D g=(Graphics2D)getGraphics(); x2=event.getX(); y2=event.getY(); if(flag==1) { red=Integer.parseInt(jt1.getText()); green=Integer.parseInt(jt2.getText()); blue=Integer.parseInt(jt3.getText()); Color c=new Color(red,green,blue); g.setColor(c); g.draw(new Line2D.Double(x1,y1,x2,y2)); } x1=x2; y1=y2; label.setText("当前鼠标的位置:("+ x2 + "," + y2 +")"); g.dispose(); } });代码分析
时间: 2024-01-19 08:04:46 浏览: 65
这段代码添加了一个鼠标移动和拖拽事件监听器,其中的`mouseMoved`方法在鼠标移动时被触发,`mouseDragged`方法在鼠标拖拽时被触发。
在`mouseMoved`方法中,没有实现任何功能,只是空方法体。在`mouseDragged`方法中,首先获取了`Graphics2D`对象,然后获取鼠标的当前坐标`x2`和`y2`,接着判断`flag`标志,如果为1,则根据文本框中的RGB值设置画笔颜色,绘制一条直线,然后更新`x1`和`y1`的值为当前坐标,更新界面上的标签显示当前鼠标位置,最后释放`Graphics2D`对象。
需要注意的是,这段代码中的绘制操作并不是线程安全的,因此在实际开发中需要注意线程安全问题。
相关问题
import javax.swing.*; import java.awt.*; import java.awt.event.*; public class DrawingBoard extends JPanel implements MouseMotionListener, MouseListener { private int lastX, lastY; public DrawingBoard() { setPreferredSize(new Dimension(400, 400)); setBackground(Color.WHITE); addMouseMotionListener(this); addMouseListener(this); } public void paintComponent(Graphics g) { super.paintComponent(g); Font font = new Font("Arial", Font.BOLD, 20); g.setFont(font); g.setColor(Color.BLUE); g.drawString("Hello World!", 50, 50); } public void mouseDragged(MouseEvent e) { Graphics g = getGraphics(); g.drawLine(lastX, lastY, e.getX(), e.getY()); lastX = e.getX(); lastY = e.getY(); } public void mousePressed(MouseEvent e) { lastX = e.getX(); lastY = e.getY(); } public void mouseMoved(MouseEvent e) {} public void mouseClicked(MouseEvent e) {} public void mouseEntered(MouseEvent e) {} public void mouseExited(MouseEvent e) {} public void mouseReleased(MouseEvent e) {} public static void main(String[] args) { JFrame frame = new JFrame("Drawing Board"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(new DrawingBoard()); frame.pack(); frame.setVisible(true); } }加注释
下面是对这段 Java 代码的注释:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
// DrawingBoard 类继承了 JPanel 类并实现了 MouseMotionListener 和 MouseListener 接口
public class DrawingBoard extends JPanel implements MouseMotionListener, MouseListener {
private int lastX, lastY; // 上一次鼠标移动的位置
// 构造函数
public DrawingBoard() {
setPreferredSize(new Dimension(400, 400)); // 设置 DrawingBoard 的首选大小
setBackground(Color.WHITE); // 设置 DrawingBoard 的背景颜色为白色
addMouseMotionListener(this); // 添加鼠标移动事件监听器
addMouseListener(this); // 添加鼠标事件监听器
}
// 重写 JPanel 的 paintComponent 方法,在 DrawingBoard 上绘制字符串
public void paintComponent(Graphics g) {
super.paintComponent(g); // 先调用父类的 paintComponent 方法
Font font = new Font("Arial", Font.BOLD, 20); // 创建字体对象
g.setFont(font); // 设置字体
g.setColor(Color.BLUE); // 设置画笔颜色为蓝色
g.drawString("Hello World!", 50, 50); // 在 DrawingBoard 上绘制字符串
}
// 鼠标拖动事件监听器
public void mouseDragged(MouseEvent e) {
Graphics g = getGraphics(); // 获取 DrawingBoard 上的画笔对象
g.drawLine(lastX, lastY, e.getX(), e.getY()); // 绘制直线,连接上一次鼠标移动的位置和当前位置
lastX = e.getX(); // 更新上一次鼠标移动的位置
lastY = e.getY();
}
// 鼠标按下事件监听器
public void mousePressed(MouseEvent e) {
lastX = e.getX(); // 记录上一次鼠标移动的位置
lastY = e.getY();
}
// 鼠标移动事件监听器(不需要实现)
public void mouseMoved(MouseEvent e) {}
// 鼠标单击事件监听器(不需要实现)
public void mouseClicked(MouseEvent e) {}
// 鼠标进入 DrawingBoard 事件监听器(不需要实现)
public void mouseEntered(MouseEvent e) {}
// 鼠标离开 DrawingBoard 事件监听器(不需要实现)
public void mouseExited(MouseEvent e) {}
// 鼠标释放事件监听器(不需要实现)
public void mouseReleased(MouseEvent e) {}
// 主函数
public static void main(String[] args) {
JFrame frame = new JFrame("Drawing Board"); // 创建 JFrame 对象
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // 设置关闭操作
frame.getContentPane().add(new DrawingBoard()); // 在 JFrame 上添加 DrawingBoard
frame.pack(); // 调整 JFrame 的大小以适应 DrawingBoard
frame.setVisible(true); // 显示 JFrame
}
}
```
public class JavaMain { public static void main(String[] args) { new MyWindow(); } } class MyWindow extends JFrame implements MouseListener, MouseMotionListener, ActionListener { //定义两个点 private Point oldPoint = null,newPoint = null; public MyWindow () { this.setSize(600,600); addMouseListener(this); addMouseMotionListener(this); this.setVisible(true); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } //画图 public void paint(Graphics g) { Graphics2D g2 = (Graphics2D) g; g2.setStroke(new BasicStroke(10.0f)); g2.setColor(Color.red); if(oldPoint!=null&&newPoint!=null) { g2.drawLine(oldPoint.x, oldPoint.y, newPoint.x, newPoint.y); oldPoint=newPoint; newPoint=null;//置为空,为了迎接下一个点的到来 } } //鼠标拖动事件 @Override public void mouseDragged(MouseEvent e) { if(oldPoint==null) { oldPoint=e.getPoint(); }else { newPoint=e.getPoint(); repaint();//生成新的点,通知系统去更新绘图 } } @Override public void mouseMoved(MouseEvent e) {} //鼠标单击事件 @Override public void mouseClicked(MouseEvent e) {} @Override public void mouseEntered(MouseEvent e) {} @Override public void mouseExited(MouseEvent e) {} @Override public void mousePressed(MouseEvent e) {} //鼠标释放事件,清空工作 @Override public void mouseReleased(MouseEvent e) { newPoint=null; oldPoint=null; } }设置清除画布按钮
您可以在 `MyWindow` 类中添加一个按钮,并为其添加一个 `ActionListener`,在按钮被点击时清除画布。代码示例如下:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class MyWindow extends JFrame implements MouseListener, MouseMotionListener, ActionListener {
private Point oldPoint = null, newPoint = null;
private JButton clearButton; // 添加一个清除按钮
public MyWindow() {
this.setSize(600, 600);
addMouseListener(this);
addMouseMotionListener(this);
// 初始化清除按钮
clearButton = new JButton("清除画布");
clearButton.addActionListener(this);
JPanel buttonPanel = new JPanel();
buttonPanel.add(clearButton);
add(buttonPanel, BorderLayout.SOUTH);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void paint(Graphics g) {
Graphics2D g2 = (Graphics2D) g;
g2.setStroke(new BasicStroke(10.0f));
g2.setColor(Color.red);
if (oldPoint != null && newPoint != null) {
g2.drawLine(oldPoint.x, oldPoint.y, newPoint.x, newPoint.y);
oldPoint = newPoint;
newPoint = null;
}
}
@Override
public void mouseDragged(MouseEvent e) {
if (oldPoint == null) {
oldPoint = e.getPoint();
} else {
newPoint = e.getPoint();
repaint();
}
}
@Override
public void mouseMoved(MouseEvent e) {}
@Override
public void mouseClicked(MouseEvent e) {}
@Override
public void mouseEntered(MouseEvent e) {}
@Override
public void mouseExited(MouseEvent e) {}
@Override
public void mousePressed(MouseEvent e) {}
@Override
public void mouseReleased(MouseEvent e) {
newPoint = null;
oldPoint = null;
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == clearButton) {
Graphics g = getGraphics();
g.setColor(getBackground());
g.fillRect(0, 0, getWidth(), getHeight());
}
}
}
```
在上面的代码中,我们添加了一个 `clearButton` 按钮,并在其上添加了一个 `ActionListener`。当按钮被点击时,会调用 `actionPerformed` 方法清除画布。在 `actionPerformed` 方法中,我们获取了 `MyWindow` 的 `Graphics` 对象,并使用 `fillRect` 方法覆盖整个画布。这样就达到了清除画布的效果。
阅读全文
相关推荐
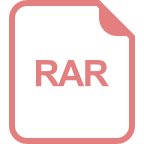
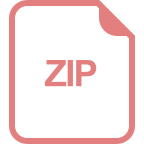
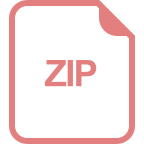













