用C++和esayx写扫雷游戏
时间: 2024-06-08 18:05:16 浏览: 95
以下是一个用C++和EasyX库实现的扫雷游戏示例:
```cpp
#include <graphics.h>
#include <conio.h>
#include <time.h>
const int BLOCK_SIZE = 20; // 方块大小
const int COLS = 30; // 列数
const int ROWS = 16; // 行数
const int BOMB_COUNT = 99; // 地雷数量
int map[ROWS][COLS]; // 地图
bool visible[ROWS][COLS]; // 是否可见
bool flag[ROWS][COLS]; // 是否标记为地雷
int uncoveredCount = 0; // 已经揭开的方块数量
// 初始化地图
void initMap() {
memset(map, 0, sizeof(map));
memset(visible, false, sizeof(visible));
memset(flag, false, sizeof(flag));
uncoveredCount = 0;
srand(time(NULL));
for (int i = 0; i < BOMB_COUNT; i++) {
int x, y;
do {
x = rand() % ROWS;
y = rand() % COLS;
} while (map[x][y] == 9); // 如果已经是地雷了就重新生成
map[x][y] = 9; // 标记为地雷
for (int dx = -1; dx <= 1; dx++) {
for (int dy = -1; dy <= 1; dy++) {
int nx = x + dx, ny = y + dy;
if (nx >= 0 && nx < ROWS && ny >= 0 && ny < COLS && map[nx][ny] != 9) {
map[nx][ny]++; // 增加周围地雷数量
}
}
}
}
}
// 绘制方块
void drawBlock(int x, int y, int val) {
if (visible[x][y]) { // 可见的方块
setfillcolor(WHITE);
fillrectangle(y * BLOCK_SIZE, x * BLOCK_SIZE, (y + 1) * BLOCK_SIZE, (x + 1) * BLOCK_SIZE);
if (val > 0) {
settextcolor(BLACK);
char buf[2];
buf[0] = val + '0';
buf[1] = '\0';
outtextxy(y * BLOCK_SIZE + 5, x * BLOCK_SIZE + 3, buf); // 绘制数字
}
} else if (flag[x][y]) { // 标记为地雷的方块
setfillcolor(RED);
fillrectangle(y * BLOCK_SIZE, x * BLOCK_SIZE, (y + 1) * BLOCK_SIZE, (x + 1) * BLOCK_SIZE);
} else { // 未知的方块
setfillcolor(LIGHTGRAY);
fillrectangle(y * BLOCK_SIZE, x * BLOCK_SIZE, (y + 1) * BLOCK_SIZE, (x + 1) * BLOCK_SIZE);
}
}
// 绘制地图
void drawMap() {
cleardevice();
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
drawBlock(i, j, map[i][j]);
}
}
}
// 揭开方块
void uncoverBlock(int x, int y) {
if (map[x][y] == 9) { // 点到了地雷
settextcolor(RED);
outtextxy(0, ROWS * BLOCK_SIZE + 3, "Oops! You clicked on a bomb!");
Sleep(1000);
initMap();
drawMap();
return;
}
visible[x][y] = true;
uncoveredCount++;
if (uncoveredCount == ROWS * COLS - BOMB_COUNT) { // 所有非地雷方块都被揭开了
settextcolor(GREEN);
outtextxy(0, ROWS * BLOCK_SIZE + 3, "Congratulations! You win!");
Sleep(1000);
initMap();
drawMap();
return;
}
if (map[x][y] == 0) { // 如果是空白方块,继续递归揭开周围方块
for (int dx = -1; dx <= 1; dx++) {
for (int dy = -1; dy <= 1; dy++) {
int nx = x + dx, ny = y + dy;
if (nx >= 0 && nx < ROWS && ny >= 0 && ny < COLS && !visible[nx][ny]) {
uncoverBlock(nx, ny);
}
}
}
}
}
// 标记为地雷或取消标记
void toggleFlag(int x, int y) {
flag[x][y] = !flag[x][y];
}
int main() {
initgraph(COLS * BLOCK_SIZE, ROWS * BLOCK_SIZE + 20);
setbkcolor(LIGHTGRAY);
settextcolor(BLACK);
settextstyle(14, 0, "Consolas");
initMap();
drawMap();
while (true) {
int x = -1, y = -1;
while (true) { // 等待鼠标点击
if (MouseHit()) {
MOUSEMSG msg = GetMouseMsg();
if (msg.uMsg == WM_LBUTTONDOWN) {
x = msg.y / BLOCK_SIZE;
y = msg.x / BLOCK_SIZE;
break;
} else if (msg.uMsg == WM_RBUTTONDOWN) {
toggleFlag(msg.y / BLOCK_SIZE, msg.x / BLOCK_SIZE);
drawBlock(msg.y / BLOCK_SIZE, msg.x / BLOCK_SIZE, map[msg.y / BLOCK_SIZE][msg.x / BLOCK_SIZE]);
}
}
}
uncoverBlock(x, y);
drawMap();
}
closegraph();
return 0;
}
```
这段代码实现了一个基本的扫雷游戏,包括地图的生成、方块的显示、鼠标事件的处理等。可以编译运行,进行游戏体验。
阅读全文
相关推荐





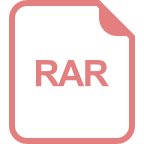



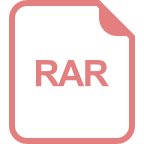

