用c++做一个扫雷游戏
时间: 2023-03-26 15:02:47 浏览: 97
好的,我可以回答这个问题。要用 C 语言编写扫雷游戏,需要使用图形库来实现游戏界面,同时需要设计算法来实现游戏逻辑。可以使用随机数生成雷区,然后根据玩家的点击来判断是否踩雷或者插旗。还需要实现计时器和计分器等功能。希望这个回答能够帮到你。
相关问题
用c++创造一个扫雷游戏
以下是用C++实现一个简单的扫雷游戏的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
using namespace std;
const int SIZE = 10;
const int NUM_MINES = 10;
void printBoard(const vector<vector<char>>& board) {
for (int i = 0; i < SIZE; i++) for (int j = 0; j < SIZE; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
void placeMines(vector<vector<char>>& board) {
int count = 0;
while (count < NUM_MINES) {
int row = rand() % SIZE;
int col = rand() % SIZE;
if (board[row][col] != '*') {
board[row][col] = '*';
count++;
}
}
}
void calculateNumbers(vector<vector<char>>& board) {
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (board[i][j] != '*') {
int count = 0;
if (i > 0 && j > 0 && board[i - 1][j - 1] == '*') {
count++;
}
if (i > 0 && board[i - 1][j] == '*') {
count++;
}
if (i > 0 && j < SIZE - 1 && board[i - 1][j + 1] == '*') {
count++;
}
if (j > 0 && board[i][j - 1] == '*') {
count++;
}
if (j < SIZE - 1 && board[i][j + 1] == '*') {
count++;
}
if (i < SIZE - 1 && j > 0 && board[i + 1][j - 1] == '*') {
count++;
}
if (i < SIZE - 1 && board[i + 1][j] == '*') {
count++;
}
if (i < SIZE - 1 && j < SIZE - 1 && board[i + 1][j + 1] == '*') {
count++;
}
board[i][j] = count + '0';
}
}
}
}
int main() {
srand(time(0));
vector<vector<char>> board(SIZE, vector<char>(SIZE, '0'));
placeMines(board);
calculateNumbers(board);
printBoard(board);
return 0;
}
```
用C++写一个扫雷游戏
好的,这是一个简单的扫雷游戏示例。首先,我们需要定义一个存储地图信息的二维数组,其中 '*' 表示地雷,'.' 表示空地。我们还需要定义一个表示地图大小的常量 `MAP_SIZE`。
```c
#define MAP_SIZE 10
char map[MAP_SIZE][MAP_SIZE] = {
{'.', '.', '*', '.', '.', '.', '.', '.', '.', '.'},
{'.', '.', '.', '.', '.', '.', '.', '.', '.', '.'},
{'.', '.', '.', '.', '.', '.', '.', '.', '.', '.'},
{'.', '.', '.', '.', '.', '.', '.', '.', '.', '.'},
{'.', '.', '.', '.', '.', '.', '.', '.', '.', '.'},
{'.', '.', '.', '.', '.', '.', '.', '.', '.', '.'},
{'.', '.', '.', '.', '.', '.', '.', '.', '.', '.'},
{'.', '.', '.', '.', '.', '.', '.', '.', '.', '.'},
{'.', '.', '.', '.', '.', '.', '.', '.', '.', '.'},
{'.', '.', '.', '.', '.', '.', '.', '.', '.', '.'}
};
```
然后,我们可以编写一个函数来计算给定位置周围的地雷数量。该函数应该接受两个参数:地图和给定位置的坐标。它应该返回一个整数,表示给定位置周围的地雷数量。
```c
int count_mines(char map[MAP_SIZE][MAP_SIZE], int x, int y) {
int count = 0;
for (int i = x - 1; i <= x + 1; i++) {
for (int j = y - 1; j <= y + 1; j++) {
if (i >= 0 && i <
阅读全文
相关推荐
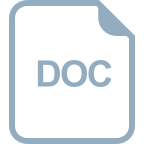
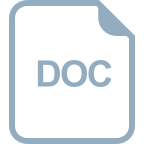
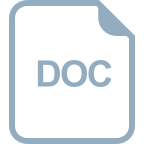







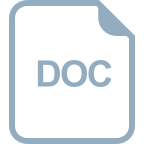
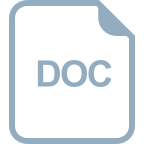
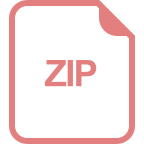
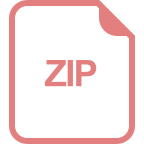