class目录下的led控制代码
时间: 2024-03-27 20:39:04 浏览: 78
在Linux系统中,使用GPIO控制LED灯可以通过访问/sys/class/gpio目录下的文件来实现。下面是一个示例代码,演示了如何通过GPIO控制LED灯。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#define LED_PIN 17
int main()
{
int fd, ret;
// 导出GPIO引脚
fd = open("/sys/class/gpio/export", O_WRONLY);
if (fd < 0) {
perror("open export");
return -1;
}
ret = write(fd, "17", 2);
if (ret < 0) {
perror("write export");
return -1;
}
close(fd);
// 设置GPIO引脚为输出模式
fd = open("/sys/class/gpio/gpio17/direction", O_WRONLY);
if (fd < 0) {
perror("open direction");
return -1;
}
ret = write(fd, "out", 3);
if (ret < 0) {
perror("write direction");
return -1;
}
close(fd);
// 循环控制LED灯
while (1) {
// 点亮LED灯
fd = open("/sys/class/gpio/gpio17/value", O_WRONLY);
if (fd < 0) {
perror("open value");
return -1;
}
ret = write(fd, "1", 1);
if (ret < 0) {
perror("write value");
return -1;
}
close(fd);
usleep(500000); // 等待500ms
// 关闭LED灯
fd = open("/sys/class/gpio/gpio17/value", O_WRONLY);
if (fd < 0) {
perror("open value");
return -1;
}
ret = write(fd, "0", 1);
if (ret < 0) {
perror("write value");
return -1;
}
close(fd);
usleep(500000); // 等待500ms
}
// 取消GPIO引脚的导出
fd = open("/sys/class/gpio/unexport", O_WRONLY);
if (fd < 0) {
perror("open unexport");
return -1;
}
ret = write(fd, "17", 2);
if (ret < 0) {
perror("write unexport");
return -1;
}
close(fd);
return 0;
}
```
在上述代码中,首先需要将LED灯连接到GPIO17引脚。然后使用open()函数打开/sys/class/gpio/export文件,并将"17"字符串写入其中,从而导出GPIO17引脚。接着使用open()函数打开/sys/class/gpio/gpio17/direction文件,并将"out"字符串写入其中,将GPIO17引脚设置为输出模式。然后进入一个循环,每次循环先使用open()函数打开/sys/class/gpio/gpio17/value文件,并将"1"字符串写入其中,从而点亮LED灯;然后等待500ms;再使用open()函数打开/sys/class/gpio/gpio17/value文件,并将"0"字符串写入其中,从而关闭LED灯;最后再等待500ms。当程序终止时,需要使用open()函数打开/sys/class/gpio/unexport文件,并将"17"字符串写入其中,从而取消GPIO17引脚的导出。
阅读全文
相关推荐
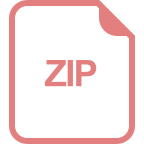
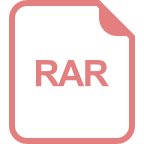
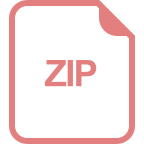
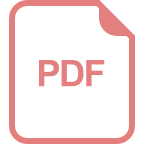
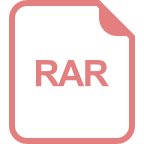
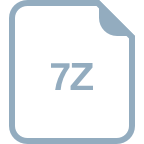
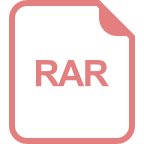
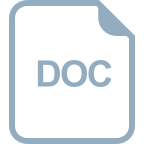
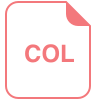
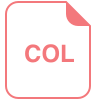





