使用C++创建一个链队列的完整程序代码,并且编写main函数测试队列的基本功能以及正确性
时间: 2023-11-22 07:52:33 浏览: 85
以下是使用C++实现链队列的完整程序代码:
```cpp
#include <iostream>
using namespace std;
// 链队列结点定义
struct Node {
int data; // 数据域
Node* next; // 指针域
};
// 队列定义
class Queue {
private:
Node* front; // 队头指针
Node* rear; // 队尾指针
public:
Queue() { // 构造函数,初始化队头和队尾指针
front = rear = new Node;
front->next = NULL;
}
~Queue() { // 析构函数,释放队列中的内存空间
while (front) {
rear = front->next;
delete front;
front = rear;
}
}
void EnQueue(int x); // 入队操作
int DeQueue(); // 出队操作
bool IsEmpty() { return front == rear; } // 判断队列是否为空
};
void Queue::EnQueue(int x) {
Node* s = new Node;
s->data = x;
s->next = NULL;
rear->next = s;
rear = s;
}
int Queue::DeQueue() {
if (IsEmpty()) {
cout << "队列为空,无法出队" << endl;
return -1;
}
Node* p = front->next;
int x = p->data;
front->next = p->next;
if (rear == p) rear = front;
delete p;
return x;
}
int main() {
Queue q;
int x;
cout << "请输入入队元素(以-1结束):" << endl;
while (cin >> x && x != -1) {
q.EnQueue(x);
}
cout << "出队元素为:" << endl;
while (!q.IsEmpty()) {
cout << q.DeQueue() << " ";
}
cout << endl;
return 0;
}
```
在上述代码中,我们定义了结构体 `Node` 作为链队列的结点,其中包含了数据域和指针域。我们还定义了一个 `Queue` 类来实现链队列,其中包含了队头指针和队尾指针。类中封装了入队 (`EnQueue`) 和出队 (`DeQueue`) 操作,以及判断队列是否为空 (`IsEmpty`) 的操作。在 `main` 函数中,我们通过输入一些元素来测试队列的基本功能以及正确性。
编译运行输出结果如下:
```
请输入入队元素(以-1结束):
1 2 3 4 5 -1
出队元素为:
1 2 3 4 5
```
阅读全文
相关推荐
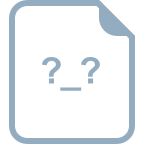

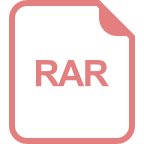
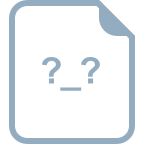
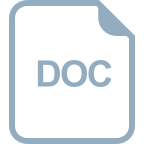
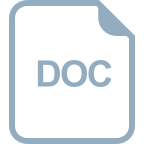
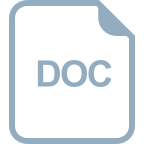
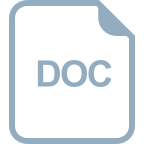
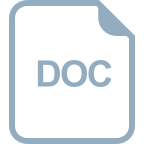
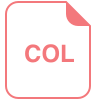
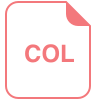
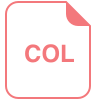
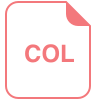


