用c++语言分支限界法解决旅行售货员
时间: 2023-12-14 15:04:39 浏览: 42
好的,下面我将为你提供一个c++语言实现的分支限界法解决旅行售货员问题的示例代码。
首先,我们需要定义一个节点类,用来表示每个状态。这个类需要包含以下属性:当前已访问的城市集合、当前路径长度、当前所在城市、当前还未访问的城市集合。
```c++
class Node {
public:
set<int> visited; // 已访问的城市集合
int path_length; // 当前路径长度
int current_city; // 当前所在城市
set<int> not_visited; // 还未访问的城市集合
Node(set<int> v, int p, int c, set<int> n) : visited(v), path_length(p), current_city(c), not_visited(n) {}
};
```
接下来,我们需要定义一个比较函数,用来对节点进行排序。这里我们使用了一个lambda表达式来定义比较函数,按照路径长度从小到大排序。
```c++
auto cmp = [](const Node &a, const Node &b) {
return a.path_length > b.path_length;
};
```
然后,我们就可以编写分支限界法的主函数了。这个函数需要接受一个邻接矩阵和城市数量作为输入,返回一个最短路径和路径顺序。
在主函数中,我们需要定义一个优先队列和一个初始节点。初始节点的已访问城市集合中只包含起点,还未访问城市集合包含除起点外的所有城市。
```c++
pair<int, vector<int>> tsp(vector<vector<int>> &graph, int n) {
priority_queue<Node, vector<Node>, decltype(cmp)> q(cmp);
set<int> visited = {0};
set<int> not_visited;
for (int i = 1; i < n; ++i) {
not_visited.insert(i);
}
Node start(visited, 0, 0, not_visited);
q.push(start);
int shortest_path = INT_MAX;
vector<int> shortest_order;
```
接下来,我们进入主循环,不断从队列中取出节点进行扩展,直到队列为空。
在扩展节点时,我们需要生成所有可能的子节点,并计算它们的路径长度。如果当前路径长度已经大于最短路径,则放弃这个节点。
```c++
while (!q.empty()) {
Node node = q.top();
q.pop();
if (node.path_length >= shortest_path) {
continue;
}
if (node.not_visited.empty()) {
shortest_path = node.path_length + graph[node.current_city][0];
shortest_order.clear();
shortest_order.push_back(0);
for (auto city : node.visited) {
shortest_order.push_back(city);
}
continue;
}
for (auto city : node.not_visited) {
set<int> new_visited = node.visited;
new_visited.insert(city);
set<int> new_not_visited = node.not_visited;
new_not_visited.erase(city);
Node new_node(new_visited, node.path_length + graph[node.current_city][city], city, new_not_visited);
q.push(new_node);
}
}
```
最后,我们返回最短路径和路径顺序。
```c++
return make_pair(shortest_path, shortest_order);
```
完整代码如下:
```c++
#include <iostream>
#include <vector>
#include <set>
#include <queue>
#include <climits>
using namespace std;
class Node {
public:
set<int> visited;
int path_length;
int current_city;
set<int> not_visited;
Node(set<int> v, int p, int c, set<int> n) : visited(v), path_length(p), current_city(c), not_visited(n) {}
};
auto cmp = [](const Node &a, const Node &b) {
return a.path_length > b.path_length;
};
pair<int, vector<int>> tsp(vector<vector<int>> &graph, int n) {
priority_queue<Node, vector<Node>, decltype(cmp)> q(cmp);
set<int> visited = {0};
set<int> not_visited;
for (int i = 1; i < n; ++i) {
not_visited.insert(i);
}
Node start(visited, 0, 0, not_visited);
q.push(start);
int shortest_path = INT_MAX;
vector<int> shortest_order;
while (!q.empty()) {
Node node = q.top();
q.pop();
if (node.path_length >= shortest_path) {
continue;
}
if (node.not_visited.empty()) {
shortest_path = node.path_length + graph[node.current_city][0];
shortest_order.clear();
shortest_order.push_back(0);
for (auto city : node.visited) {
shortest_order.push_back(city);
}
continue;
}
for (auto city : node.not_visited) {
set<int> new_visited = node.visited;
new_visited.insert(city);
set<int> new_not_visited = node.not_visited;
new_not_visited.erase(city);
Node new_node(new_visited, node.path_length + graph[node.current_city][city], city, new_not_visited);
q.push(new_node);
}
}
return make_pair(shortest_path, shortest_order);
}
int main() {
int n = 4;
vector<vector<int>> graph(n, vector<int>(n, 0));
graph[0][1] = 10;
graph[0][2] = 15;
graph[0][3] = 20;
graph[1][0] = 5;
graph[1][2] = 9;
graph[1][3] = 10;
graph[2][0] = 6;
graph[2][1] = 13;
graph[2][3] = 12;
graph[3][0] = 8;
graph[3][1] = 8;
graph[3][2] = 9;
auto res = tsp(graph, n);
cout << "Shortest path: " << res.first << endl;
cout << "Path order: ";
for (auto city : res.second) {
cout << city << " ";
}
cout << endl;
return 0;
}
```
这个代码片段中,我使用了一个邻接矩阵来表示城市之间的距离,以及一个4个城市的例子来测试代码的正确性。你可以根据自己的需求修改这些参数。
相关推荐
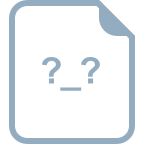
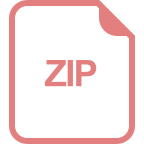














