fstream
时间: 2023-11-09 09:12:51 浏览: 33
`fstream` 是C++中的一个标准库,用于在文件中进行读写操作。它是 `ifstream` 和 `ofstream` 的组合,可以实现同时读写文件的功能。
使用 `fstream`,我们可以打开一个文件,读取其中的数据并进行操作,也可以将数据写入文件中。例如,我们可以使用以下代码创建一个 `fstream` 对象并打开一个文件:
```
#include <fstream>
#include <iostream>
int main() {
std::fstream file("example.txt");
if (!file) {
std::cout << "File not found." << std::endl;
return 1;
}
// 在这里可以进行读写操作
file.close();
return 0;
}
```
在这个示例中,我们尝试打开名为 `example.txt` 的文件。如果文件不存在,我们会输出一个错误消息并退出程序。如果成功打开文件,我们可以在 `if` 语句中执行读写操作,然后使用 `close()` 函数关闭文件。
相关问题
c fstream
C++ 中的 fstream 是用于文件输入输出操作的类。它包含在 `<fstream>` 头文件中。
通过 fstream 类,我们可以打开文件并进行读写操作。它提供了基于文本和二进制的输入输出功能。
下面是一个简单的示例代码,演示了如何使用 fstream 来读取和写入文件:
```cpp
#include <iostream>
#include <fstream>
int main() {
std::ofstream outfile("example.txt"); // 创建一个输出文件流对象
if (outfile.is_open()) {
outfile << "Hello, C++ fstream!"; // 写入数据到文件
outfile.close(); // 关闭文件流
}
else {
std::cout << "Failed to open the file." << std::endl;
}
std::ifstream infile("example.txt"); // 创建一个输入文件流对象
if (infile.is_open()) {
std::string content;
getline(infile, content); // 从文件中读取一行数据
std::cout << "Content: " << content << std::endl;
infile.close(); // 关闭文件流
}
else {
std::cout << "Failed to open the file." << std::endl;
}
return 0;
}
```
在这个示例中,我们首先创建了一个 `ofstream` 对象 `outfile` 来打开一个名为 `example.txt` 的文件,并向其中写入一行数据。然后,我们关闭了文件流。
接下来,我们使用 `ifstream` 对象 `infile` 来打开同样的文件,并从中读取一行数据到一个字符串变量中。最后,我们关闭了文件流。
这只是 fstream 类的一些基本用法,你可以根据实际需求进一步探索和使用它提供的各种功能。
cpp fstream
在C++中,可以使用fstream库来进行文件的输入和输出操作。fstream库提供了三个主要的类:ifstream(用于输入操作)、ofstream(用于输出操作)和fstream(用于输入和输出操作)。
要使用fstream库,需要包含<fstream>头文件。下面是一个简单的示例,演示了如何使用fstream进行文件的读写操作:
```cpp
#include <iostream>
#include <fstream>
int main() {
// 文件写入操作
std::ofstream outfile("example.txt"); // 创建一个输出文件流对象
if (outfile.is_open()) { // 检查文件是否成功打开
outfile << "Hello, World!" << std::endl; // 写入数据到文件
outfile.close(); // 关闭文件流
} else {
std::cout << "Unable to open file for writing." << std::endl;
}
// 文件读取操作
std::ifstream infile("example.txt"); // 创建一个输入文件流对象
if (infile.is_open()) { // 检查文件是否成功打开
std::string line;
while (std::getline(infile, line)) { // 逐行读取文件内容
std::cout << line << std::endl; // 输出每一行内容
}
infile.close(); // 关闭文件流
} else {
std::cout << "Unable to open file for reading." << std::endl;
}
return 0;
}
```
以上代码演示了如何使用fstream进行文件的写入和读取操作。在示例中,首先创建一个ofstream对象outfile,开名为"example.txt"的文件并写入数据。接着创建一个ifstream对象infile,打开同样的文件
相关推荐
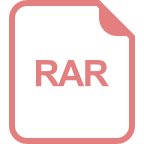
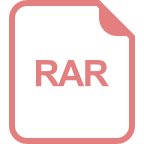
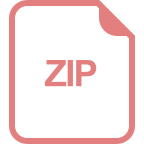











