C++ fstream
时间: 2023-11-09 16:56:27 浏览: 142
`fstream` 是 C++ 中用于文件输入输出的类。它是通过将 `ifstream`(用于文件输入)和 `ofstream`(用于文件输出)结合起来形成的。
要使用 `fstream` 类进行文件操作,需要包含头文件 `<fstream>`。下面是一些常见的 `fstream` 操作示例:
1. 打开文件并写入数据:
```cpp
#include <fstream>
int main() {
std::ofstream file("example.txt"); // 打开文件 example.txt 进行写入
if (file.is_open()) {
file << "Hello, World!"; // 写入数据到文件
file.close(); // 关闭文件
} else {
// 处理文件打开失败的情况
}
return 0;
}
```
2. 从文件中读取数据:
```cpp
#include <fstream>
#include <iostream>
#include <string>
int main() {
std::ifstream file("example.txt"); // 打开文件 example.txt 进行读取
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl; // 输出每一行数据
}
file.close(); // 关闭文件
} else {
// 处理文件打开失败的情况
}
return 0;
}
```
在上面的示例中,使用 `ofstream` 对象来打开文件并进行写入操作,使用 `ifstream` 对象来打开文件并进行读取操作。通过调用 `is_open()` 函数来检查文件是否成功打开。使用 `<<` 操作符来写入数据,使用 `getline()` 函数来逐行读取文件数据。
需要注意的是,文件操作完成后应该调用 `close()` 函数来关闭文件。另外,还可以使用一些其他的 `fstream` 成员函数来进行更复杂的文件操作,如移动文件指针、判断文件是否到达文件末尾等。
阅读全文
相关推荐
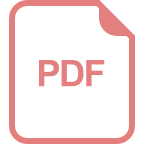



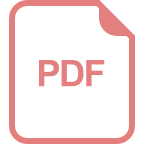
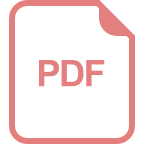
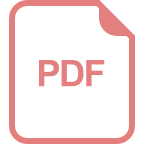
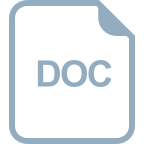








