JAVA红球1到33选6个,蓝球1到16选1个,红球每颗不能重复,实现自动生成中奖号码,控制台输入自选号码,进行号码比对,根据下注数输出中了多少奖。简单代码
时间: 2024-12-12 07:33:03 浏览: 4
在Java中,你可以创建一个随机生成彩票号码的方法,并结合用户输入的自选号码来进行比对。以下是一个简单的示例代码:
```java
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Random;
public class LotteryGenerator {
public static void main(String[] args) {
// 创建红球列表
List<Integer> redBalls = new ArrayList<>(Arrays.asList(1, 2, ..., 33));
// 创建蓝球列表
List<Integer> blueBall = Arrays.asList(1, 2, ..., 16);
// 确保红球不重复
Random random = new Random();
List<Integer> selectedRedBalls = new ArrayList<>();
while (selectedRedBalls.size() < 6) {
int ball = random.nextInt(redBalls.size());
if (!selectedRedBalls.contains(ball)) {
selectedRedBalls.add(ball);
redBalls.remove(ball);
}
}
// 选择蓝球
int selectedBlueBall = random.nextInt(blueBall.size());
System.out.println("自动产生的中奖号码:红球 " + selectedRedBalls + ", 蓝球 " + selectedBlueBall);
// 用户自选号码
List<Integer> userNumbers = getUserInput();
// 比对并输出结果
int matchedBalls = compareNumbers(selectedRedBalls, selectedBlueBall, userNumbers);
System.out.println("您下注的号码与中奖号码相比:匹配了" + matchedBalls + "个号码。");
}
// 获取用户输入的号码
private static List<Integer> getUserInput() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入您的自选红球号码(6个数字,用逗号分隔):");
String input = scanner.nextLine();
return Arrays.asList(Integer.parseInt(num) for num in input.split(","));
}
// 比较两个列表的相同元素个数
private static int compareNumbers(List<Integer> generated, int blue, List<Integer> user) {
int count = 0;
for (int userNum : user) {
if (generated.contains(userNum)) {
count++;
}
}
return count;
}
}
阅读全文
相关推荐
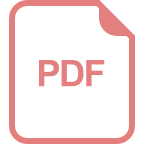
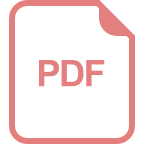
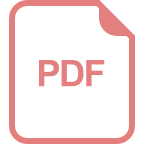















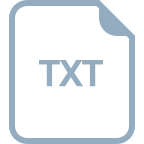
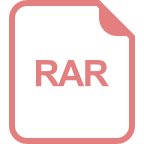