残差来实现图像特征提取功能,Python实现完整带码
时间: 2024-09-19 07:11:33 浏览: 34
残差网络(Residual Networks, ResNet)是一种深度学习模型,在卷积神经网络(CNN)中引入了跳跃连接(skip connection),使得网络能够有效地解决梯度消失的问题,从而可以训练更深的网络,提高图像特征提取的能力。在图像特征提取方面,残差块的设计允许信息直接从输入传递到输出,这对于捕捉长距离依赖至关重要。
在Python中,特别是使用Keras或PyTorch库实现ResNet,通常需要以下步骤:
1. **安装依赖**:
```bash
pip install tensorflow keras torchvision (对于TensorFlow用户)
or
pip install torch torchvision (对于PyTorch用户)
```
2. **导入必要的模块**:
```python
from tensorflow.keras.layers import Conv2D, BatchNormalization, Activation, Add, Input, MaxPooling2D
# 或者
import torch.nn as nn
```
3. **定义残差块**(Residual Block):
- Keras示例:
```python
def residual_block(x, filters, strides=1):
shortcut = x
if strides != 1 or x.shape[-1] != filters:
shortcut = Conv2D(filters, kernel_size=1, strides=strides)(x)
x = Conv2D(filters, kernel_size=3, padding='same', strides=strides)(x)
x = BatchNormalization()(x)
x = Activation('relu')(x)
x = Conv2D(filters, kernel_size=3, padding='same')(x)
x = BatchNormalization()(x)
x = Add()([shortcut, x])
return x
```
- PyTorch示例:
```python
class ResidualBlock(nn.Module):
def __init__(self, in_channels, out_channels, stride=1):
super().__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=3, stride=stride, padding=1)
self.bn1 = nn.BatchNorm2d(out_channels)
self.relu = nn.ReLU(inplace=True)
self.conv2 = nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.bn2 = nn.BatchNorm2d(out_channels)
self.shortcut = nn.Sequential()
if stride != 1 or in_channels != out_channels:
self.shortcut = nn.Sequential(
nn.Conv2d(in_channels, out_channels, kernel_size=1, stride=stride),
nn.BatchNorm2d(out_channels)
)
def forward(self, x):
out = self.conv1(x)
out = self.bn1(out)
out = self.relu(out)
out = self.conv2(out)
out = self.bn2(out)
out += self.shortcut(x) # skip connection
out = self.relu(out)
return out
```
4. **构建完整的ResNet模型**:
- 示例略,可以根据需要设置层数、通道数等参数,并添加适当的池化层和全连接层用于分类或其他任务。
5. **编译并训练模型**:
```python
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(X_train, y_train, validation_data=(X_val, y_val), epochs=epochs, batch_size=batch_size)
```
阅读全文
相关推荐
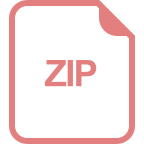
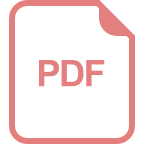
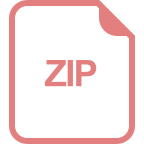

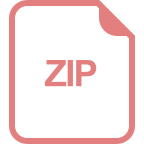
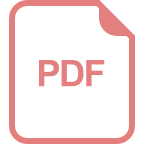
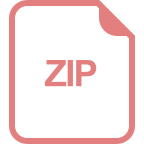
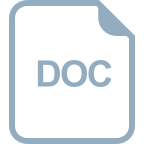
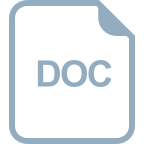
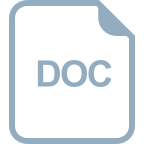
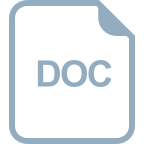
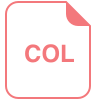
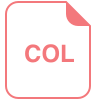
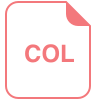
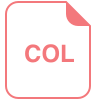
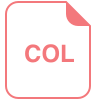


