c++中priority_queue decltype
时间: 2023-08-08 15:11:06 浏览: 107
在C++中,`priority_queue`是一个容器适配器,它提供了一个优先级队列的功能,其中元素按照特定的优先级进行排序。`priority_queue`可以在头文件`<queue>`中找到。
`decltype`是C++11引入的一个关键字,用于根据表达式的类型推断出变量的类型。可以在编译时确定表达式的类型,而无需显式指定。
当使用`decltype`和`priority_queue`结合时,可以使用`decltype`推断出`priority_queue`中元素的类型。例如:
```cpp
#include <queue>
int main() {
std::priority_queue<int> pq;
using Element = decltype(pq)::value_type; // 使用decltype推断出元素的类型
// 其他操作...
return 0;
}
```
在上面的示例中,使用`decltype(pq)::value_type`可以推断出`priority_queue`中元素的类型为`int`。
相关问题
priority_queue decltype
priority_queue是一个C++ STL容器,用于实现优先队列。在声明priority_queue对象时,可以使用decltype关键字指定Compare类型。
引用中给出了两种使用decltype的示例。第一种使用了cmpFun的指针作为Compare类型,第二种使用了cmpFun的引用作为Compare类型。
另一种常见的方法是使用仿函数对优先队列进行自定义排序。在声明priority_queue对象时,需要显式地定义Container类型和Compare类型。引用中给出了一个使用仿函数的示例,其中Node是元素类型,vector<Node>是容器类型,Cmp是Compare类型。
如果使用lambda表达式进行自定义排序,则不需要使用decltype。引用中提到,可以通过重载Node类型的<运算符来满足less<Node>的要求。
因此,当声明priority_queue对象时,可以使用decltype来指定Compare类型,也可以使用仿函数或lambda表达式来进行自定义排序。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [[STL]priority_queue多种方式自定义排序](https://blog.csdn.net/Strengthennn/article/details/119078911)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
c++定义priority_queue的比较函数
在 C++ 中,可以使用 `std::priority_queue` 来定义优先队列。要定义比较函数,你可以使用函数指针、函数对象或者 Lambda 表达式。下面是几种不同的方法:
1. 使用函数指针:
```cpp
bool compare(int a, int b) {
// 自定义的比较逻辑,返回 true 表示 a 在 b 之前
// 返回 false 表示 a 在 b 之后
// 例如,按照升序排列:
return a > b;
}
std::priority_queue<int, std::vector<int>, bool (*)(int, int)> pq(compare);
```
2. 使用函数对象(重载 `operator()`):
```cpp
struct Compare {
bool operator()(int a, int b) {
// 自定义的比较逻辑,返回 true 表示 a 在 b 之前
// 返回 false 表示 a 在 b 之后
// 例如,按照升序排列:
return a > b;
}
};
std::priority_queue<int, std::vector<int>, Compare> pq;
```
3. 使用 Lambda 表达式:
```cpp
auto compare = [](int a, int b) {
// 自定义的比较逻辑,返回 true 表示 a 在 b 之前
// 返回 false 表示 a 在 b 之后
// 例如,按照升序排列:
return a > b;
};
std::priority_queue<int, std::vector<int>, decltype(compare)> pq(compare);
```
无论使用哪种方法,都需要确保比较函数能够正确判断元素的优先级。在创建 `std::priority_queue` 对象时,将比较函数作为第三个模板参数传递给它。
阅读全文
相关推荐
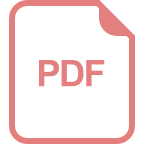
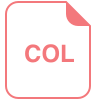

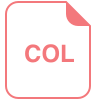
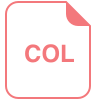









