#include <stdio.h> #include<stdlib.h> struct student { int num; char name[20]; int score; }; int main( ) { int i; struct student st,stmax,stmin; stmax.score=0; stmin.score=100; printf("\n input data"); for(i=0;i<10;i++) { scanf("%d%s%d",&st.num,st.name,&st.score); if(st.score>stmax.score) stmax=st; if(st.score<stmin.score) stmin=st; } printf("\n hight:%5d%15s%5d",stmax.num,stmax.name,stmax.score); printf("\n low:%5d%15s%5d",stmin.num,stmin.name,stmin.score); system("pause"); return 0; }分析一下
时间: 2024-03-08 10:44:54 浏览: 68
这个程序主要是通过结构体来存储学生信息,并使用循环来输入10个学生的信息,然后在输入过程中判断当前学生的分数是否比当前最高或最低分数高或低,如果是则更新最高或最低分数学生的信息。最后输出最高和最低分数学生的信息。
具体来说,程序中定义了一个结构体`student`,包含三个成员变量,分别是学号、姓名和分数。在主函数中,定义了三个结构体变量,分别用来存储当前分数最高、最低的学生和当前输入的学生。然后使用循环输入10个学生的信息,并在输入过程中判断当前学生的分数是否比当前最高或最低分数高或低,如果是则更新最高或最低分数学生的信息。最后输出最高和最低分数学生的信息。
需要注意的是,程序中使用了`system("pause")`来让程序暂停等待用户输入,这种写法在不同的操作系统和编译环境下可能会有不同的效果。
相关问题
#include <stdio.h> #include <stdlib.h> #include <string.h> #define MAX_STUDENTS 100 // 最多支持100个学生 struct date{ int year; int month; int day; }; typedef struct { int num; // 学号 char name[20]; // 姓名 char sex[5]; // 性别 struct date birthday; // 出生日期 int score[3]; // 三门功课成绩 int total; // 总分 double average; // 平均分 } student; student students[MAX_STUDENTS]; // 学生数组 int num_students = 0; // 学生数量
这段代码定义了一个名为student的结构体,其中包含了学生的学号、姓名、性别、出生日期、三门功课成绩、总分和平均分等信息。同时还定义了一个名为date的结构体,用来表示学生的出生日期。
另外还定义了一个名为students的数组来存储学生信息,数组的长度为MAX_STUDENTS,即最多支持100个学生。还定义了一个变量num_students用来记录当前学生数量。
这段代码的作用是定义了一些数据结构和变量,用来存储和处理学生信息。具体的操作需要在其他代码中实现。
#include <stdio.h> #include <stdlib.h> #include <string.h> #define MAX_SIZE 100 // 学生信息结构体 typedef struct { char num[10]; // 学号 char name[10]; // 姓名 char clas[10]; // 班级 char sex[10]; // 性别 float score; // 成绩 } Student; // 学生信息数组 Student studentList[MAX_SIZE]; int size = 0; 帮我添加一个可以查询学生成绩最大值和最小值的分函数代码
可以添加以下代码来实现查询学生成绩最大值和最小值的函数:
```c
// 查询学生成绩最大值和最小值
void findMinMaxScore() {
float maxScore = 0, minScore = 100;
for (int i = 0; i < size; i++) {
if (studentList[i].score > maxScore) {
maxScore = studentList[i].score;
}
if (studentList[i].score < minScore) {
minScore = studentList[i].score;
}
}
printf("成绩最高的学生信息:\n");
for (int i = 0; i < size; i++) {
if (studentList[i].score == maxScore) {
printf("学号:%s 姓名:%s 班级:%s 性别:%s 成绩:%.2f\n",
studentList[i].num, studentList[i].name,
studentList[i].clas, studentList[i].sex,
studentList[i].score);
}
}
printf("成绩最低的学生信息:\n");
for (int i = 0; i < size; i++) {
if (studentList[i].score == minScore) {
printf("学号:%s 姓名:%s 班级:%s 性别:%s 成绩:%.2f\n",
studentList[i].num, studentList[i].name,
studentList[i].clas, studentList[i].sex,
studentList[i].score);
}
}
}
```
在主函数中,可以通过调用该函数来查询学生成绩最大值和最小值:
```c
int main() {
// 添加学生信息
// ...
// 查询学生成绩最大值和最小值
findMinMaxScore();
return 0;
}
```
阅读全文
相关推荐
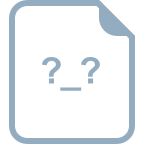














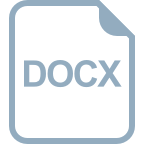