python中凯撒加密程序设计
时间: 2023-11-06 14:08:46 浏览: 86
好的,关于Python中的凯撒加密程序设计,可以使用以下代码实现:
```python
def caesar_cipher(text, shift):
result = ""
for i in range(len(text)):
char = text[i]
if char.isupper():
result += chr((ord(char) + shift - 65) % 26 + 65)
else:
result += chr((ord(char) + shift - 97) % 26 + 97)
return result
```
这个函数可以接收两个参数:待加密的文本和偏移量。它将每个字符的ASCII码加上偏移量,然后将结果转换回字符形式。如果字符是大写字母,则加上65;如果是小写字母,则加上97。最后,将加密后的文本作为函数的返回值。
请问还有什么问题需要我回答吗?
相关问题
用python 自己编写程序,测试字符串相应方法,及正则表达式,完成凯撒加密程序设计
好的,我可以帮你回答这个问题。下面是一个简单的凯撒加密程序的设计,使用Python语言实现。
```python
def caesar_encrypt(plaintext, shift):
"""
凯撒加密函数
plaintext: 明文
shift: 加密偏移量
"""
ciphertext = ""
for char in plaintext:
if char.isalpha():
# 对于字母字符进行加密
if char.islower():
ciphertext += chr((ord(char) - 97 + shift) % 26 + 97)
else:
ciphertext += chr((ord(char) - 65 + shift) % 26 + 65)
else:
# 对于非字母字符原样输出
ciphertext += char
return ciphertext
```
上面这个函数接受两个参数,明文和加密偏移量,返回加密后的密文。它的实现方法是对明文中的每个字符进行加密,对于字母字符应用凯撒加密算法,对于非字母字符原样输出。这里假设明文只包含英文字母和空格符。
下面是一个简单的测试例子:
```python
plaintext = "hello world"
shift = 3
ciphertext = caesar_encrypt(plaintext, shift)
print("明文:", plaintext)
print("密文:", ciphertext)
```
输出结果为:
```
明文: hello world
密文: khoor zruog
```
你还可以使用Python的正则表达式模块re来进行字符串的匹配和替换操作。例如,要将一个字符串中的所有数字替换成星号,可以使用如下代码:
```python
import re
s = "abc123def456"
s = re.sub(r"\d", "*", s)
print(s)
```
输出结果为:
```
abc***def***
```
上面的代码中,re.sub函数使用正则表达式r"\d"匹配字符串中的所有数字,然后将其替换成星号。
请你用Python编写程序测试字符串相应方法 及正则表达式,完成凯撒加密程序设计
好的,下面是一个Python程序,演示了字符串方法和正则表达式的使用,以及凯撒加密的实现:
```python
import re
# 字符串方法示例程序
s = "Hello, World!"
print("原始字符串:", s)
print("大写字母:", s.upper())
print("小写字母:", s.lower())
print("列表:", s.split(","))
if s.startswith("Hello"):
print("字符串以'Hello'开头")
else:
print("字符串不以'Hello'开头")
if s.endswith("World!"):
print("字符串以'World!'结尾")
else:
print("字符串不以'World!'结尾")
print("子字符串'World'的位置:", s.find("World"))
print("替换后的字符串:", s.replace("World", "Python"))
# 正则表达式示例程序
pattern = r'\d+' # 匹配一个或多个数字
text = 'hello 123 world 456'
match = re.search(pattern, text)
if match:
print("匹配到的数字是:", match.group())
else:
print("没有匹配到数字")
# 凯撒加密程序设计
def caesar_cipher(s, k):
result = ""
for c in s:
if c.isalpha(): # 只加密字母
if c.isupper():
result += chr((ord(c) + k - 65) % 26 + 65) # 大写字母
else:
result += chr((ord(c) + k - 97) % 26 + 97) # 小写字母
else:
result += c # 非字母字符直接保留
return result
# 测试凯撒加密程序
plaintext = "Hello, World!"
key = 3
ciphertext = caesar_cipher(plaintext, key)
print("加密前:", plaintext)
print("加密后:", ciphertext)
```
这个程序演示了字符串方法和正则表达式的使用,以及凯撒加密的实现。在凯撒加密的实现中,我们定义了一个函数`caesar_cipher`来进行加密操作,它接受两个参数,分别是明文和密钥。在函数内部,我们遍历明文中的每个字符,对于字母字符,根据其大小写进行加密,对于非字母字符直接保留,最后返回加密后的密文。
希望这个程序能够帮助您更好地理解字符串方法和正则表达式的使用,并且帮助您实现凯撒加密。
阅读全文
相关推荐
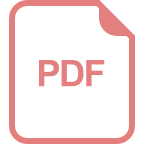
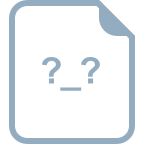
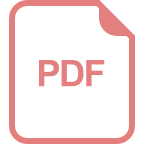
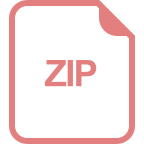
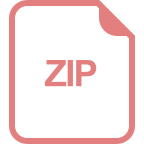
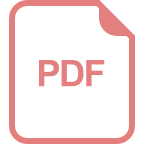
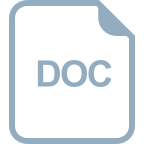
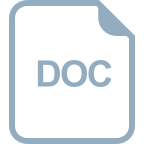
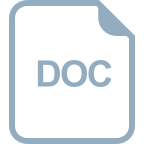






