设计编写程序,测试字符串相应方法,及正则表达式,完成凯撒加密程序设计
时间: 2024-03-10 22:44:20 浏览: 20
好的,我可以帮您设计编写这个程序。下面是一个基本的凯撒加密程序的实现过程:
```python
def caesar_cipher(text, shift):
"""
凯撒加密算法实现函数
:param text: 需要加密的文本
:param shift: 加密偏移量
:return: 加密后的文本
"""
cipher_text = ""
for char in text:
if char.isalpha():
# 处理字母加密
if char.isupper():
cipher_text += chr((ord(char) - 65 + shift) % 26 + 65)
else:
cipher_text += chr((ord(char) - 97 + shift) % 26 + 97)
else:
# 处理非字母字符
cipher_text += char
return cipher_text
```
`caesar_cipher` 函数接受两个参数,分别是需要加密的文本和加密偏移量。函数会处理文本中的每个字符,对字母进行加密,对非字母字符进行保留。加密后的字符会拼接成一个新的字符串并返回。
下面是一个简单的测试代码:
```python
text = "Hello, World!"
shift = 3
cipher_text = caesar_cipher(text, shift)
print(cipher_text)
```
输出结果为:
```
Khoor, Zruog!
```
接下来,我们可以测试一些字符串的方法和正则表达式。例如,我们可以测试字符串的 `upper` 和 `lower` 方法:
```python
text = "Hello, World!"
print(text.upper()) # 输出 HELLO, WORLD!
print(text.lower()) # 输出 hello, world!
```
还可以使用正则表达式来匹配、查找和替换字符串。例如,我们可以使用 `re` 模块来查找文本中的数字:
```python
import re
text = "Hello, 123456 World!"
pattern = r"\d+"
matches = re.findall(pattern, text)
print(matches) # 输出 ['123456']
```
以上就是一个简单的凯撒加密程序和一些字符串方法和正则表达式的示例。希望能对您有所帮助。
相关推荐
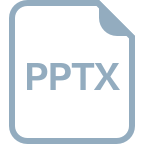
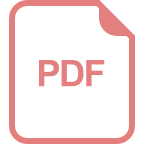
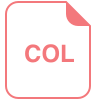
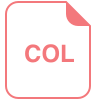
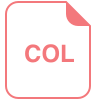
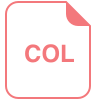
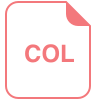









