用python 自己编写程序,测试字符串相应方法,及正则表达式,完成凯撒加密程序设计
时间: 2024-03-09 09:46:10 浏览: 72
好的,我可以帮你回答这个问题。下面是一个简单的凯撒加密程序的设计,使用Python语言实现。
```python
def caesar_encrypt(plaintext, shift):
"""
凯撒加密函数
plaintext: 明文
shift: 加密偏移量
"""
ciphertext = ""
for char in plaintext:
if char.isalpha():
# 对于字母字符进行加密
if char.islower():
ciphertext += chr((ord(char) - 97 + shift) % 26 + 97)
else:
ciphertext += chr((ord(char) - 65 + shift) % 26 + 65)
else:
# 对于非字母字符原样输出
ciphertext += char
return ciphertext
```
上面这个函数接受两个参数,明文和加密偏移量,返回加密后的密文。它的实现方法是对明文中的每个字符进行加密,对于字母字符应用凯撒加密算法,对于非字母字符原样输出。这里假设明文只包含英文字母和空格符。
下面是一个简单的测试例子:
```python
plaintext = "hello world"
shift = 3
ciphertext = caesar_encrypt(plaintext, shift)
print("明文:", plaintext)
print("密文:", ciphertext)
```
输出结果为:
```
明文: hello world
密文: khoor zruog
```
你还可以使用Python的正则表达式模块re来进行字符串的匹配和替换操作。例如,要将一个字符串中的所有数字替换成星号,可以使用如下代码:
```python
import re
s = "abc123def456"
s = re.sub(r"\d", "*", s)
print(s)
```
输出结果为:
```
abc***def***
```
上面的代码中,re.sub函数使用正则表达式r"\d"匹配字符串中的所有数字,然后将其替换成星号。
阅读全文
相关推荐
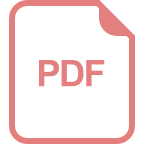
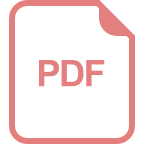
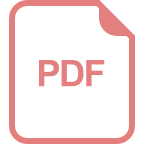


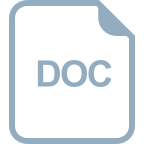
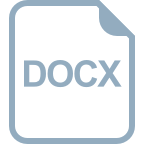
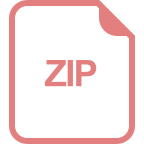
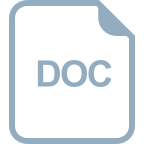
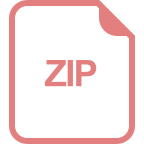
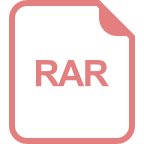
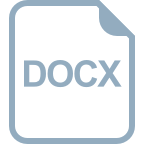
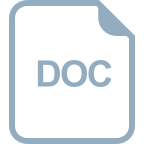
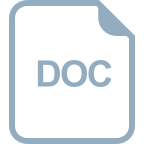
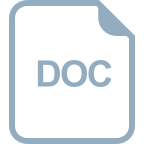
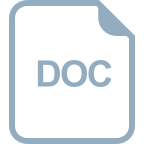
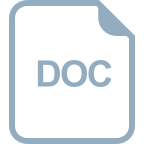
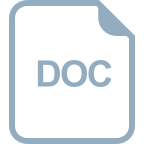
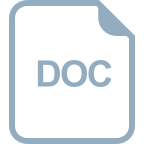