使用Python编写一个程序实现凯撒加密
时间: 2024-12-10 20:50:10 浏览: 26
在Python中,凯撒加密是一种简单易懂的替换式密码技术,通过将明文中的每个字符按照固定的偏移量向右移动来达到加密目的。下面是一个基本的凯撒加密的实现示例:
```python
def caesar_cipher(plaintext, shift):
encrypted_text = ""
for char in plaintext:
if char.isalpha():
# 对字母区分大小写
shifted_char = chr(((ord(char.lower()) - ord('a' if char.islower() else 'A')) + shift) % 26 + ord('a' if char.islower() else 'A'))
encrypted_text += shifted_char
else:
# 非字母字符不变
encrypted_text += char
return encrypted_text
# 示例:将明文 "Hello, World!" 加密,偏移量为3
shift = 3
plaintext = "Hello, World!"
encrypted_text = caesar_cipher(plaintext, shift)
print(f"原始明文:{plaintext}")
print(f"加密后的文本:{encrypted_text}")
相关问题
python编写程序,实现凯撒密码加密算法。
Python是一种简单易学的编程语言,它具有广泛的应用领域。凯撒密码是一种简单的加密算法,它通过将字母按照一定的偏移量进行替换来实现加密。
下面是使用Python编写凯撒密码加密算法的示例代码:
```python
def caesar_encrypt(text, shift):
encrypted_text = ""
for char in text:
if char.isalpha():
ascii_offset = ord('A') if char.isupper() else ord('a')
encrypted_char = chr((ord(char) - ascii_offset + shift) % 26 + ascii_offset)
encrypted_text += encrypted_char
else:
encrypted_text += char
return encrypted_text
# 测试
text = "Hello, World!"
shift = 3
encrypted_text = caesar_encrypt(text, shift)
print("加密后的文本:", encrypted_text)
```
在上面的代码中,`caesar_encrypt`函数接受两个参数:`text`表示要加密的文本,`shift`表示偏移量。函数首先遍历文本中的每个字符,如果是字母,则根据其大小写确定ASCII码的偏移量。然后,通过将字符的ASCII码减去偏移量,并对26取模,得到加密后的字符的ASCII码。最后,将加密后的字符拼接到`encrypted_text`字符串中。最终,函数返回加密后的文本。
以上是使用Python编写凯撒密码加密算法的示例代码。如果你有任何问题,请随时提问。
编写一个凯撒加密的python程序
好的,我会尽力为您解答。以下是一段简单的Python程序,实现了凯撒加密:
```python
def caesar_cipher(message, key):
ciphertext = ""
for char in message:
if char.isalpha():
if char.isupper():
ciphertext += chr((ord(char) - 65 + key) % 26 + 65)
else:
ciphertext += chr((ord(char) - 97 + key) % 26 + 97)
else:
ciphertext += char
return ciphertext
```
以上程序中,`message` 参数表示需要加密的明文消息,`key` 参数表示加密使用的密钥,类型为整数。程序首先遍历明文消息中的每个字符,如果是字母则使用凯撒密码进行加密,非字母字符则直接保留。该程序支持大小写字母的加密,并且将非字母字符原样保留。
使用该程序进行加密时,只需调用 `caesar_cipher` 函数并传入两个参数,如下所示:
```python
plaintext = "hello world"
key = 3
ciphertext = caesar_cipher(plaintext, key)
print(ciphertext)
```
此时输出的结果为:`khoor zruog`。这就是使用凯撒密码对明文消息进行加密后得到的密文。值得注意的是,该加密方式并不安全,容易被破解,仅适用于简单的保密需求。
阅读全文
相关推荐
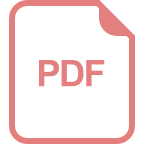
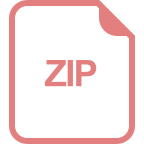
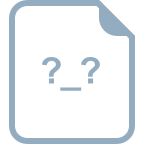













