python:编写加密函数,使用凯撒加密方法对字符串加密
时间: 2024-01-25 17:07:03 浏览: 85
好的,我可以帮您解答这个问题。以下是一个用Python实现的凯撒加密函数:
```python
def caesar_encrypt(text, key):
"""使用凯撒加密方法对字符串进行加密"""
encrypted_text = ""
for char in text:
if char.isalpha():
shifted_char = chr((ord(char) + key - 97) % 26 + 97)
encrypted_text += shifted_char
else:
encrypted_text += char
return encrypted_text
```
其中,`text`参数是需要加密的字符串,`key`参数是加密使用的密钥。这个函数将字符串中的每个字母进行移位操作来实现加密。
例如,如果密钥为2,那么字母A将被替换成C,字母B将被替换成D,以此类推。
请注意,凯撒加密并不是一种安全的加密方式,并且很容易被破解。因此,在实际应用中,需要使用更加安全的加密方式,比如AES加密算法。
相关问题
python编写加密函数,使用凯撒加密方法对字符串加密。
好的,关于Python编写加密函数,可以使用凯撒加密方法对字符串进行加密。凯撒加密是一种基于移位的加密方法,将明文中的每个小写字母都移动指定的位数来得到密文。以下是一个简单的Python程序,实现凯撒加密方法:
```
def caesar_encrypt(plaintext, shift):
ciphertext = ""
for c in plaintext:
if c.isalpha():
if c.islower():
ciphertext += chr((ord(c) - 97 + shift) % 26 + 97)
else:
ciphertext += chr((ord(c) - 65 + shift) % 26 + 65)
else:
ciphertext += c
return ciphertext
```
使用该函数,将字符串进行加密很简单,比如:
```
text = "Hello, world!"
shift = 3
encrypted_text = caesar_encrypt(text, shift)
print("Plaintext: ", text)
print("Encrypted text: ", encrypted_text)
```
输出结果为:
```
Plaintext: Hello, world!
Encrypted text: Khoor, zruog!
```
希望对您有帮助!
用python编写加密函数,使用凯撒加密方法对字符串加密
好的,我可以帮您回答这个问题。以下是一个使用凯撒加密方法对字符串进行加密的 Python 函数示例:
```python
def caesar_cipher(text, shift):
result = ""
for char in text:
if char.isalpha():
# 获取该字母在字母表中的位置
index = ord(char.lower()) - 97
# 加上偏移量并取模
new_index = (index + shift) % 26
# 获取加密后的字母
new_char = chr(new_index + 97)
# 根据原始字母是否大写,决定是否将新字母转为大写
if char.isupper():
new_char = new_char.upper()
# 拼接到结果字符串中
result += new_char
else:
# 对于非字符的字符,直接拼接到结果字符串中
result += char
return result
```
该函数接受两个参数:要加密的文本和偏移量。它通过将每个字母在字母表中移动指定的偏移量,从而对文本进行凯撒加密。如果想要解密,只需要将偏移量取相反数即可。如果您想要尝试更高级的加密方法,例如 AES 或 DES,您可以参考 Python 的标准库中的 `cryptography` 模块。
阅读全文
相关推荐
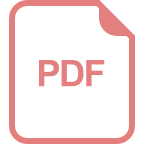
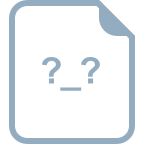




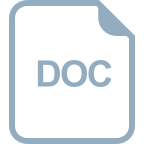









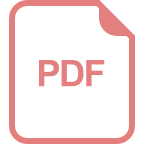