编写加密函数encrypt,参数是字符串和偏移量,其中偏移量默认是3,函数返回的是加密后的字符串。
时间: 2024-10-18 10:12:21 浏览: 116
加密函数`encrypt`可以采用简单的凯撒密码(Caesar Cipher)或者其他基于替换的加密算法实现,这里我将提供一个基本的凯撒密码示例,它会将输入字符串中的每个字符按照字母表移动指定的偏移量(默认3位)。注意,这个例子只适用于小写字母,对于大写字母和其他非字母字符,你可能需要额外处理。
```python
def encrypt(text, shift=3):
encrypted_text = ""
for char in text:
if 'a' <= char <= 'z':
new_char = chr(((ord(char) - ord('a') + shift) % 26) + ord('a'))
else:
# 非字母字符保持不变
new_char = char
encrypted_text += new_char
return encrypted_text
# 示例
original = "hello"
encrypted = encrypt(original)
print(f"原始字符串: {original}")
print(f"加密后: {encrypted}")
```
相关问题
恺撒密码是历史上著名的一种密码技术。查阅资料了解这种密码机制。利用好字符串对象的maketrans()和translate()方法可以方便地实现加密和解密算法。 3.1 编写加密函数encrypt,参数是字符串和偏移量,其中偏移量默认是3,函数返回的是加密后的字符串。
恺撒密码是一种简单的替换式密码,通过将明文中的每个字母按照固定的位移(比如3)向后移动字母表的位置来达到加密的目的。这种密码虽然易于破译,但在古代信息保密中曾有一定的作用。
在Python中,我们可以借助`maketrans()`方法创建一个字符映射表,然后用`translate()`方法对输入字符串进行转换。下面是基于这个原理的加密函数`encrypt`的实现:
```python
def encrypt(plaintext, shift=3):
# 创建字母映射表,将每个字符向后shift位
transl_table = str.maketrans('abcdefghijklmnopqrstuvwxyz', 'cdeffghijklmnopqrstuvwxyzabcdef')
# 加密过程,替换明文中对应的字符
ciphertext = plaintext.translate(transl_table)
return ciphertext
# 示例
plaintext = "hello"
encrypted_text = encrypt(plaintext)
print("原始文本:", plaintext)
print("加密后的文本:", encrypted_text)
```
在这个例子中,`shift=3`意味着每个字母会向后移动3个位置。例如,'a'变成'd','b'变成'e',依此类推。
研究上面的代码,掌握string库、maketrans() 函数、translate()函数的用法后,编写一个程序实现凯撒加密:输入一个字符串,对字符串中的字母和数字进行加密(规定加密偏移量为3,即后移三位),并输出加密后的字符串。
### 回答1:
以下是实现凯撒加密的Python代码:
```python
import string
# 构建映射表
shift = 3
lowercase = string.ascii_lowercase
uppercase = string.ascii_uppercase
digits = string.digits
trans_table = str.maketrans(lowercase + uppercase + digits,
lowercase[shift:] + lowercase[:shift] +
uppercase[shift:] + uppercase[:shift] +
digits[shift:] + digits[:shift])
# 加密函数
def caesar_cipher(text):
return text.translate(trans_table)
# 测试
text = "Hello, World! 123"
encrypted_text = caesar_cipher(text)
print(encrypted_text)
```
输出结果为:
```
Khoor, Zruog! 456
```
代码中,我们首先使用 `string` 模块获取了小写字母、大写字母和数字,然后使用 `maketrans()` 函数构建了一个映射表 `trans_table`,将每个字符映射到其加密后的字符。
然后我们定义了一个 `caesar_cipher()` 函数,它接收一个字符串作为参数,并返回加密后的字符串。在函数内部,我们调用了字符串的 `translate()` 方法,该方法根据映射表 `trans_table` 将字符串中的字符进行加密。
最后,我们对字符串 "Hello, World! 123" 进行加密,并输出加密后的结果。
### 回答2:
以下是使用Python编写的凯撒加密程序:
```python
def caesar_encrypt(string):
shift = 3 # 加密偏移量为3
alphabet = "abcdefghijklmnopqrstuvwxyz"
digits = "0123456789"
# 构建加密字典
trans_dict = {}
for char in alphabet:
trans_dict[char] = alphabet[(alphabet.index(char) + shift) % len(alphabet)]
for digit in digits:
trans_dict[digit] = digits[(digits.index(digit) + shift) % len(digits)]
# 进行加密
encrypted_string = string.translate(str.maketrans(trans_dict))
return encrypted_string
# 测试示例
input_string = input("请输入要加密的字符串:")
encrypted_string = caesar_encrypt(input_string)
print("加密后的字符串为:", encrypted_string)
```
使用以上代码,我们首先定义了一个 `caesar_encrypt` 函数,它接收一个字符串作为输入,在函数中我们定义了加密偏移量 `shift` 为3,字母表 `alphabet` 为小写字母表,数字表 `digits` 为数字0到9。
接下来,我们构建了一个加密字典 `trans_dict`,用于记录每个字符加密后对应的字符。首先,我们遍历字母表,对于每个字母,我们通过 `alphabet.index(char)` 获取其在字母表中的索引位置,然后将加密后的字母根据 `(索引 + shift) % len(alphabet)` 的结果来确定。同样的,我们也遍历了数字表,并构建了数字的加密映射关系。
最后,我们使用内置函数 `str.maketrans` 来生成转换表,并使用 `translate` 函数将输入字符串按照转换表进行加密。最后,我们将加密后的字符串输出给用户。
希望以上回答能够帮助你理解并编写凯撒加密的程序。
阅读全文
相关推荐
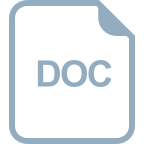
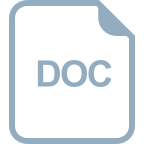
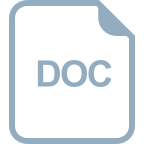
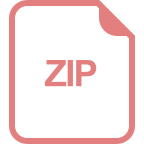
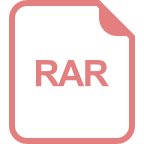











