直接插入排序、冒泡排序、简单选择写一个完整c语言代码并运行
时间: 2023-08-12 19:05:41 浏览: 92
好的,以下是直接插入排序、冒泡排序、简单选择排序的C语言代码:
直接插入排序:
```c
#include <stdio.h>
void insertion_sort(int array[], int n) {
int i, j, key;
for (i = 1; i < n; i++) {
key = array[i];
j = i - 1;
while (j >= 0 && array[j] > key) {
array[j + 1] = array[j];
j--;
}
array[j + 1] = key;
}
}
int main() {
int array[] = { 5, 2, 4, 6, 1, 3 };
int n = sizeof(array) / sizeof(array[0]);
insertion_sort(array, n);
for (int i = 0; i < n; i++) {
printf("%d ", array[i]);
}
return 0;
}
```
冒泡排序:
```c
#include <stdio.h>
void bubble_sort(int array[], int n) {
int i, j;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (array[j] > array[j + 1]) {
int temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
}
int main() {
int array[] = { 5, 2, 4, 6, 1, 3 };
int n = sizeof(array) / sizeof(array[0]);
bubble_sort(array, n);
for (int i = 0; i < n; i++) {
printf("%d ", array[i]);
}
return 0;
}
```
简单选择排序:
```c
#include <stdio.h>
void selection_sort(int array[], int n) {
int i, j, min_idx;
for (i = 0; i < n - 1; i++) {
min_idx = i;
for (j = i + 1; j < n; j++) {
if (array[j] < array[min_idx]) {
min_idx = j;
}
}
int temp = array[i];
array[i] = array[min_idx];
array[min_idx] = temp;
}
}
int main() {
int array[] = { 5, 2, 4, 6, 1, 3 };
int n = sizeof(array) / sizeof(array[0]);
selection_sort(array, n);
for (int i = 0; i < n; i++) {
printf("%d ", array[i]);
}
return 0;
}
```
你可以将这些代码复制到一个.c文件中,然后使用C语言编译器编译并运行。例如,使用gcc编译器可以使用以下命令编译:
```
gcc -o sort sort.c
```
然后运行生成的可执行文件:
```
./sort
```
输出应该如下:
```
1 2 3 4 5 6
```
阅读全文
相关推荐
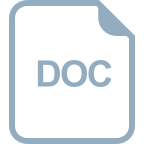
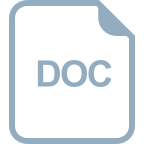
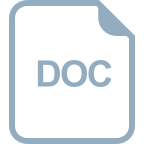
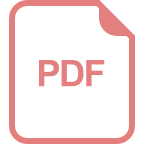














