问题描述 给定散列表的数据类型如下 typedef int ElementType; typedef struct HashNode { ElementType key; struct HashNode * next; }HashNode,* List; typedef struct { int size; List * lists;//槽指针,所有链表均有头结点 }HashTable; 请设计HashNode * find(HashTable * h,ElementType key) 该函数在散列表中查找关键字key,并返回该结点指针;如果找不到则返回NULL。 以下函数已经定义,你可以直接调用 int hash(HashTable * h,ElementType key); 请注意,本题有预置代码,只需提交所要求的函数定义代码即可。 预置代码 #include <bits/stdc++.h> using namespace std; typedef int ElementType; typedef struct HashNode { ElementType key; struct HashNode * next; }HashNode,* List; typedef struct { int size; List * lists;//槽指针,所有链表均有头结点 }HashTable; HashNode * find(HashTable * h,ElementType key); int main() { /*此处代码由测试程序自动添加,主要为了向顺序表中插入数据 并输出数据,你无需关心此处代码的具体实现细节。 如果有必要,请自己添加代码以测试你的函数是否正确。 */ return 0; } /*你的提交的代码将被添加在此处,请完成题目所要求的函数的定义*/c代码
时间: 2024-02-06 18:02:18 浏览: 120
下面是我根据题目需求编写的代码:
```c++
HashNode * find(HashTable * h, ElementType key) {
int index = hash(h, key);
HashNode * node = h->lists[index]->next;
while (node != nullptr) {
if (node->key == key) {
return node;
}
node = node->next;
}
return nullptr;
}
```
首先,我们需要根据关键字 `key` 计算出它所对应的槽下标 `index`,这可以通过调用 `hash` 函数来实现。然后,我们需要遍历该槽中的链表,查找是否存在关键字 `key`。如果找到了,我们就可以返回该结点指针。如果遍历完链表还没有找到,我们就可以返回 `nullptr`,表示找不到该关键字。
需要注意的是,由于该散列表中的每个链表都有头结点,因此我们需要从头结点的下一个结点开始遍历链表。
相关问题
给定散列表的数据类型如下 typedef int ElementType; typedef struct HashNode { ElementType key; struct HashNode * next; }HashNode,* List; typedef struct { int size; List * lists;//槽指针,所有链表均有头结点 }HashTable; 请设计int hash(HashTable * h,ElementType key) 该函数计算关键字key的散列值,本题中散列值简单的取为key对散列表容量的余数。 请注意,本题有预置代码,只需提交所要求的函数定义代码即可。 c代码
好的,根据题目要求,我可以为你设计一个计算关键字key散列值的函数hash:
```c
int hash(HashTable *h, ElementType key) {
return key % h->size;
}
```
该函数接收一个 HashTable 指针和一个 ElementType 类型的关键字 key,返回 key 对散列表容量的余数作为散列值。
怎么由typedef struct HashNode { ElementType key; struct HashNode * next; }HashNode,* List; typedef struct { int size; List * lists; //槽指针,所有链表均有头结点 }HashTable;来生成一个空的散列表
要生成一个空的散列表,可以按照以下步骤进行:
1. 定义散列表的大小,即槽的数量,可以使用一个常量来指定,例如:
```cpp
const int kHashTableSize = 100;
```
2. 分配内存空间来存储散列表的槽指针,即 `lists` 数组,可以使用 `malloc` 或 `new` 进行动态内存分配,例如:
```cpp
List * lists = new List[kHashTableSize];
```
3. 对于每个链表,使用一个头结点来表示,可以使用 `malloc` 或 `new` 分配内存空间来存储头结点,例如:
```cpp
for (int i = 0; i < kHashTableSize; i++) {
List head = new HashNode;
head->next = NULL;
lists[i] = head;
}
```
4. 最后,将散列表的大小和槽指针数组存储在一个结构体中,并返回该结构体作为空的散列表,例如:
```cpp
HashTable * createHashTable() {
HashTable * hashTable = new HashTable;
hashTable->size = kHashTableSize;
hashTable->lists = new List[kHashTableSize];
for (int i = 0; i < kHashTableSize; i++) {
List head = new HashNode;
head->next = NULL;
hashTable->lists[i] = head;
}
return hashTable;
}
```
完整的代码示例如下:
```cpp
#include <iostream>
using namespace std;
typedef int ElementType;
typedef struct HashNode {
ElementType key;
struct HashNode * next;
} HashNode, * List;
typedef struct {
int size;
List * lists; //槽指针,所有链表均有头结点
} HashTable;
const int kHashTableSize = 100;
HashTable * createHashTable() {
HashTable * hashTable = new HashTable;
hashTable->size = kHashTableSize;
hashTable->lists = new List[kHashTableSize];
for (int i = 0; i < kHashTableSize; i++) {
List head = new HashNode;
head->next = NULL;
hashTable->lists[i] = head;
}
return hashTable;
}
int main() {
HashTable * hashTable = createHashTable();
return 0;
}
```
阅读全文
相关推荐
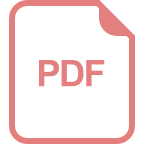
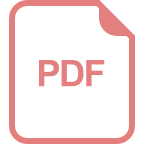
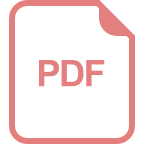
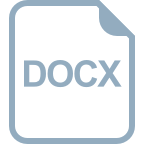
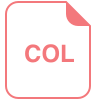

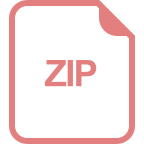
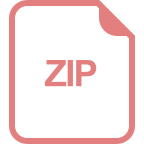
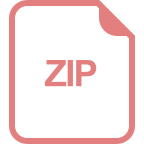
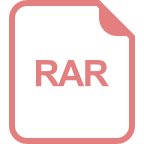
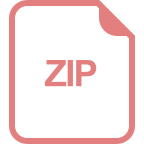
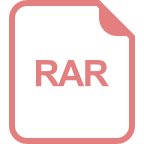