numpy中resize的用法
时间: 2023-05-28 09:06:50 浏览: 57
numpy中的resize函数用于调整数组的大小。其语法如下:
```python
numpy.resize(a, new_shape)
```
其中,a是需要调整大小的数组,new_shape是新的形状。如果new_shape大于原来的形状,则会在数组的末尾填充元素;如果new_shape小于原来的形状,则会截取数组的末尾元素。
示例:
```python
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
print(np.resize(a, (3, 2))) # 调整为3行2列的数组
```
输出结果:
```
array([[1, 2],
[3, 4],
[5, 6]])
```
相关问题
numpy resize 用法
numpy.resize() 函数可以调整数组的大小,它接受两个参数:第一个参数是要调整大小的数组,第二个参数是新的大小。如果新的大小大于原始大小,则新数组将填充默认值以扩展数组。如果新的大小小于原始大小,则原始数组的一部分将被删除以缩小数组。
下面是一个示例代码:
import numpy as np
a = np.array([[0, 1], [2, 3]])
print("原始数组:")
print(a)
b = np.resize(a, (2, 3))
print("调整大小后的数组:")
print(b)
输出结果如下:
原始数组:
[[0 1]
[2 3]]
调整大小后的数组:
[[0 1 2]
[3 0 1]]
介绍一下numpy中的reshape与resize的差别及用法
numpy中的reshape和resize都可以用于改变数组的形状,但它们之间存在一些差别。
1. reshape
reshape方法可以在不改变数组元素的情况下改变数组的形状,返回一个新的数组。其用法如下:
```
numpy.reshape(arr, newshape, order='C')
```
其中,arr表示要改变形状的数组,newshape是一个整数或者一个整数元组,表示新的形状。order参数表示新数组的存储顺序,可以为'C'或'F'。
例如:
```
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
b = np.reshape(a, (3, 2))
print(a)
print(b)
```
输出为:
```
[[1 2 3]
[4 5 6]]
[[1 2]
[3 4]
[5 6]]
```
2. resize
resize方法可以改变数组的形状,并且可以改变数组元素的数量。如果新的形状比原来的形状大,那么新数组的某些元素将被重复。如果新的形状比原来的形状小,那么新数组的某些元素将被删除。其用法如下:
```
numpy.resize(arr, new_shape)
```
其中,arr表示要改变形状的数组,new_shape是一个整数元组,表示新的形状。
例如:
```
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
b = np.resize(a, (3, 2))
print(a)
print(b)
```
输出为:
```
[[1 2 3]
[4 5 6]]
[[1 2]
[3 4]
[5 6]]
```
再例如:
```
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
b = np.resize(a, (3, 3))
print(a)
print(b)
```
输出为:
```
[[1 2 3]
[4 5 6]]
[[1 2 3]
[4 5 6]
[1 2 3]]
```
可以看到,当新形状比原来的形状大时,resize方法会重复原数组的元素。
相关推荐
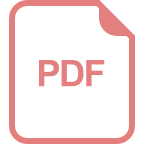
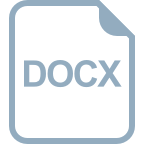
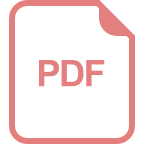












