写一个线性表结构,实现电话本模拟程序,包括电话本的建立、插入、删除、修改、逆置、查找、输出,并设计一个主菜单完成各个功能的调用。
时间: 2023-04-09 22:04:42 浏览: 91
好的,这是一个编程类的问题,我可以回答。以下是一个简单的线性表结构实现电话本模拟程序的代码:
```lua
-- 定义一个节点结构
local Node = {}
Node.__index = Node
function Node:new(name, phone)
local node = {}
setmetatable(node, Node)
node.name = name
node.phone = phone
node.next = nil
return node
end
-- 定义一个链表结构
local LinkedList = {}
LinkedList.__index = LinkedList
function LinkedList:new()
local list = {}
setmetatable(list, LinkedList)
list.head = nil
list.size = 0
return list
end
function LinkedList:insert(name, phone)
local node = Node:new(name, phone)
if self.head == nil then
self.head = node
else
node.next = self.head
self.head = node
end
self.size = self.size + 1
end
function LinkedList:delete(name)
if self.head == nil then
return
end
if self.head.name == name then
self.head = self.head.next
self.size = self.size - 1
return
end
local prev = self.head
local curr = self.head.next
while curr ~= nil do
if curr.name == name then
prev.next = curr.next
self.size = self.size - 1
return
end
prev = curr
curr = curr.next
end
end
function LinkedList:update(name, phone)
local curr = self.head
while curr ~= nil do
if curr.name == name then
curr.phone = phone
return
end
curr = curr.next
end
end
function LinkedList:reverse()
if self.head == nil or self.head.next == nil then
return
end
local prev = nil
local curr = self.head
while curr ~= nil do
local next = curr.next
curr.next = prev
prev = curr
curr = next
end
self.head = prev
end
function LinkedList:find(name)
local curr = self.head
while curr ~= nil do
if curr.name == name then
return curr.phone
end
curr = curr.next
end
return nil
end
function LinkedList:print()
local curr = self.head
while curr ~= nil do
print(curr.name, curr.phone)
curr = curr.next
end
end
-- 主程序
local phonebook = LinkedList:new()
while true do
print("1. 插入联系人")
print("2. 删除联系人")
print("3. 修改联系人")
print("4. 逆置电话本")
print("5. 查找联系人")
print("6. 输出电话本")
print("0. 退出程序")
local choice = io.read("*n")
if choice == 1 then
print("请输入联系人姓名:")
local name = io.read("*line")
print("请输入联系人电话:")
local phone = io.read("*line")
phonebook:insert(name, phone)
elseif choice == 2 then
print("请输入要删除的联系人姓名:")
local name = io.read("*line")
phonebook:delete(name)
elseif choice == 3 then
print("请输入要修改的联系人姓名:")
local name = io.read("*line")
print("请输入新的联系人电话:")
local phone = io.read("*line")
phonebook:update(name, phone)
elseif choice == 4 then
phonebook:reverse()
elseif choice == 5 then
print("请输入要查找的联系人姓名:")
local name = io.read("*line")
local phone = phonebook:find(name)
if phone ~= nil then
print(name, phone)
else
print("未找到该联系人")
end
elseif choice == 6 then
phonebook:print()
elseif choice == 0 then
break
else
print("无效的选择")
end
end
```
这个程序实现了一个简单的电话本模拟程序,可以进行联系人的插入、删除、修改、逆置、查找和输出等操作。
阅读全文
相关推荐
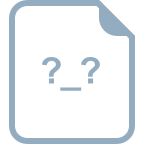

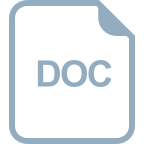
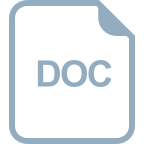






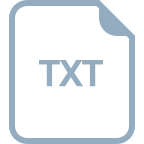
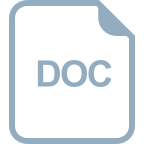




