使用python写一个使用Excel表格的内容制作词云图的代码
时间: 2024-02-23 12:01:33 浏览: 69
好的,以下是使用Python制作Excel表格内容的词云图的代码:
```python
import pandas as pd
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取Excel表格
df = pd.read_excel('data.xlsx')
# 将表格中的文本内容转换为字符串
text = ' '.join(df['text'].astype(str).tolist())
# 制作词云图
wordcloud = WordCloud(background_color="white", width=800, height=400).generate(text)
# 显示词云图
plt.figure(figsize=(12, 6))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
上述代码假定Excel表格中有一列名为“text”,其中包含需要制作词云图的文本内容。你需要将代码中的"data.xlsx"替换为你自己的Excel文件名,并确保文件路径正确。此外,你还需要安装Pandas、WordCloud和Matplotlib这三个Python库。
相关问题
python 读取excel某一列绘制词云图
Python是一种强大的编程语言,用于数据分析和可视化。要使用Python读取Excel某一列并绘制词云图,需要使用一些Python库。
首先,需要安装并导入pandas库来读取Excel文件。pandas库是一个数据处理工具,用于处理Excel表格和其他表格数据。
导入pandas库后,需要使用read_excel函数读取Excel表格,并使用iloc函数选择需要绘制词云图的某一列。
接着,安装并导入matplotlib库来绘制词云图。matplotlib是一个绘制图表的Python库。
然后,使用WordCloud库来生成词云图。WordCloud是一个Python库,用于生成词云图。
最后,使用matplotlib显示词云图。
以下是Python代码示例:
import pandas as pd
from wordcloud import WordCloud
import matplotlib.pyplot as plt
df = pd.read_excel('excel文件名.xlsx')
text = " ".join(review for review in df['列名'])
wordcloud = WordCloud(width=800, height=400, max_words=500, background_color='white').generate(text)
plt.figure(figsize=(10, 10))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
这个代码片段将读取Excel文件的某一列,将其所有行合并为一个字符串,然后使用WordCloud生成词云图并使用matplotlib显示它。
总之,Python是使用pandas、matplotlib和WordCloud等库生成词云图的最佳选择。使用这些库和代码示例,可以轻松地读取Excel某一列并绘制词云图。
将excel里面一列数据生成python词云图
好的,下面是将Excel表格中一列数据生成词云图的Python示例代码:
```python
import pandas as pd
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取Excel文件
df = pd.read_excel('data.xlsx', usecols=['text'])
# 将所有文本合并为一个字符串,用空格分隔
text = ' '.join(df['text'].astype(str))
# 创建词云对象
wordcloud = WordCloud(background_color='white', width=800, height=400).generate(text)
# 显示词云
plt.figure(figsize=(10, 10))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
其中,`data.xlsx`是包含数据的Excel文件,`usecols`参数用于指定读取的列,这里只读取了名为`text`的一列。`astype(str)`用于将该列中的所有值转换为字符串类型。可以根据需要修改词云图的参数,例如背景颜色、大小等。最后通过`plt.show()`将词云图显示出来。
相关推荐
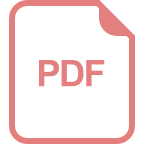
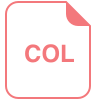
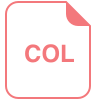
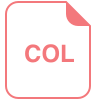









