java向量叉乘求交点
时间: 2023-07-14 20:56:50 浏览: 177
如果您想要求两条直线的交点,可以使用以下步骤:
1.定义两个向量
假设有两条直线AB和CD,首先需要定义它们的向量AB和CD。
2.计算法向量
通过向量叉乘可以得到两个向量的法向量N。
3.计算参数t
在平面几何中,两条直线的交点可以表示为两条直线上的两个点之间的参数t。可以通过以下公式计算参数t:
t = [(C - A) x N] / (B x N)
其中,A和B是向量AB的起点和终点,C和D是向量CD的起点和终点,x表示向量叉乘。
4.计算交点
通过参数t可以计算出两条直线的交点P,公式如下:
P = A + t(B - A)
其中,A和B是向量AB的起点和终点。
注意:如果两条直线平行,即N的长度为0,则它们没有交点。
相关问题
java向量叉乘求交点代码
以下是 Java 中向量叉乘求交点的示例代码:
```java
public class Vector3D {
public double x, y, z;
public Vector3D(double x, double y, double z) {
this.x = x;
this.y = y;
this.z = z;
}
// 向量叉乘
public static Vector3D crossProduct(Vector3D a, Vector3D b) {
double x = a.y * b.z - a.z * b.y;
double y = a.z * b.x - a.x * b.z;
double z = a.x * b.y - a.y * b.x;
return new Vector3D(x, y, z);
}
// 求交点
public static Vector3D getIntersectionPoint(Vector3D p1, Vector3D v1, Vector3D p2, Vector3D v2) {
Vector3D n = crossProduct(v1, v2);
Vector3D p = new Vector3D(p2.x - p1.x, p2.y - p1.y, p2.z - p1.z);
double t = crossProduct(p, v2).dotProduct(n) / v1.dotProduct(n);
double x = p1.x + v1.x * t;
double y = p1.y + v1.y * t;
double z = p1.z + v1.z * t;
return new Vector3D(x, y, z);
}
// 向量点积
public double dotProduct(Vector3D v) {
return x * v.x + y * v.y + z * v.z;
}
}
```
其中,`Vector3D` 类表示三维向量,包含三个属性 `x`、`y`、`z` 和三个方法 `crossProduct`、`getIntersectionPoint`、`dotProduct`,分别对应向量叉乘、求交点、向量点积。使用时,可以先实例化两个向量 `p1` 和 `v1`,以及另外两个向量 `p2` 和 `v2`,然后调用 `getIntersectionPoint` 方法得到它们的交点。
java二维向量叉乘求交点代码
以下是 Java 中二维向量叉乘求交点的示例代码:
```java
public class Vector2D {
public double x, y;
public Vector2D(double x, double y) {
this.x = x;
this.y = y;
}
// 向量叉乘
public static double crossProduct(Vector2D a, Vector2D b) {
return a.x * b.y - a.y * b.x;
}
// 求交点
public static Vector2D getIntersectionPoint(Vector2D p1, Vector2D v1, Vector2D p2, Vector2D v2) {
double t = crossProduct(new Vector2D(p2.x - p1.x, p2.y - p1.y), v2) / crossProduct(v1, v2);
double x = p1.x + v1.x * t;
double y = p1.y + v1.y * t;
return new Vector2D(x, y);
}
}
```
其中,`Vector2D` 类表示二维向量,包含两个属性 `x`、`y` 和两个方法 `crossProduct`、`getIntersectionPoint`,分别对应向量叉乘、求交点。使用时,可以先实例化两个向量 `p1` 和 `v1`,以及另外两个向量 `p2` 和 `v2`,然后调用 `getIntersectionPoint` 方法得到它们的交点。
阅读全文
相关推荐
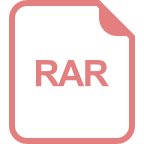
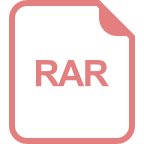
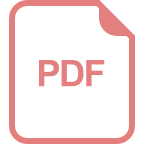
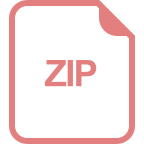
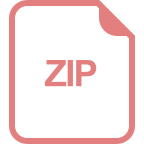
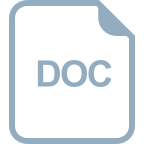
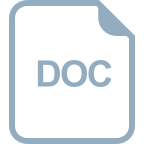
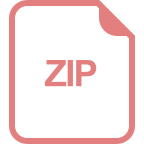
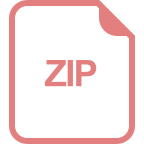
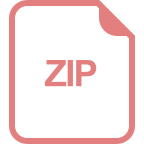
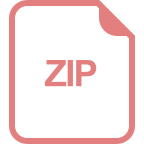
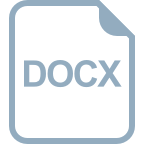
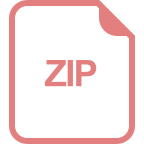
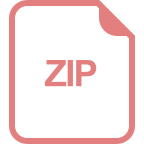
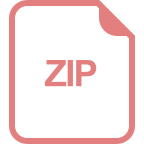
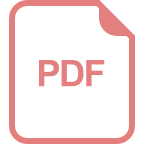