用C++写一个数据结构条件如下:使用环形队列,将字符‘a’,‘c’,‘b’,‘d’,‘f’,‘e’,‘g’依次压入队中,然后(1)输出队列中各元素的内容(2)将队尾元素出队,输出队中剩下的元素的内容(3)将字符‘k’入队
时间: 2023-09-22 18:08:15 浏览: 84
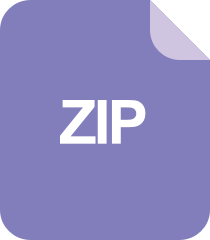
利用C++实现以下经典数据结构算法:线性表、栈、队列、数组(特殊矩阵、稀疏矩阵压缩)、串(朴素模式匹配、KMP算法)、树.zip
好的,根据您的要求,我可以为您提供一个使用环形队列实现的C++数据结构代码,如下所示:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 10; // 环形队列的最大长度
class CircularQueue {
private:
char data[MAXSIZE]; // 存储队列元素的数组
int front; // 队头指针
int rear; // 队尾指针
public:
CircularQueue() {
front = 0;
rear = 0;
}
bool isEmpty() {
return front == rear;
}
bool isFull() {
return (rear + 1) % MAXSIZE == front;
}
bool enqueue(char ch) {
if (isFull()) {
return false;
}
data[rear] = ch;
rear = (rear + 1) % MAXSIZE;
return true;
}
bool dequeue(char& ch) {
if (isEmpty()) {
return false;
}
ch = data[front];
front = (front + 1) % MAXSIZE;
return true;
}
void printQueue() {
cout << "队列中的元素为:";
int i = front;
while (i != rear) {
cout << data[i] << " ";
i = (i + 1) % MAXSIZE;
}
cout << endl;
}
};
int main() {
CircularQueue queue;
char ch;
// 将字符‘a’,‘c’,‘b’,‘d’,‘f’,‘e’,‘g’依次压入队中
queue.enqueue('a');
queue.enqueue('c');
queue.enqueue('b');
queue.enqueue('d');
queue.enqueue('f');
queue.enqueue('e');
queue.enqueue('g');
// 输出队列中各元素的内容
queue.printQueue();
// 将队尾元素出队,输出队中剩下的元素的内容
queue.dequeue(ch);
cout << "出队的元素为:" << ch << endl;
queue.printQueue();
// 将字符‘k’入队
queue.enqueue('k');
queue.printQueue();
return 0;
}
```
在这个程序中,我们使用了一个 `CircularQueue` 类来实现环形队列。在 `CircularQueue` 类中,我们使用了 `data` 数组来存储队列元素,使用 `front` 指针和 `rear` 指针来分别指向队头和队尾。
在 `enqueue(char ch)` 函数中,我们首先判断队列是否已满,如果已满则返回 false,否则将元素插入到队尾,并将 `rear` 指针向后移动一个位置。在 `dequeue(char& ch)` 函数中,我们首先判断队列是否为空,如果为空则返回 false,否则将队头元素取出并保存到 `ch` 变量中,并将 `front` 指针向后移动一个位置。
在 `printQueue()` 函数中,我们使用 `i` 变量从队头开始循环遍历整个队列,输出队列中的所有元素。
最后,在 `main()` 函数中,我们创建了一个 `CircularQueue` 对象 `queue`,将字符 ‘a’,‘c’,‘b’,‘d’,‘f’,‘e’,‘g’ 依次压入队列中,并输出队列中的所有元素。然后,我们将队尾元素出队,并输出队列中剩下的元素,接着将字符 ‘k’ 入队,并输出队列中的所有元素。
阅读全文
相关推荐
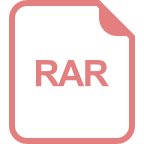
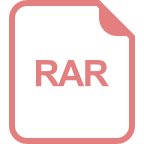
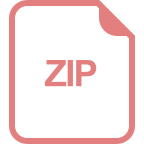
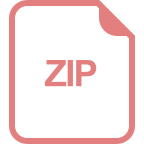
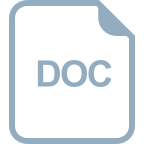
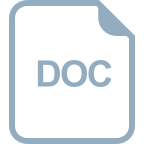
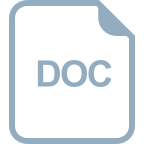
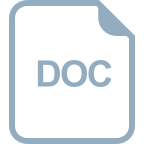
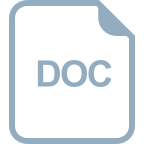
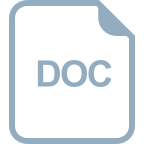
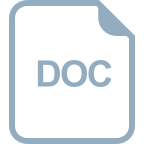
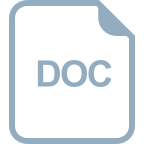
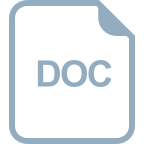
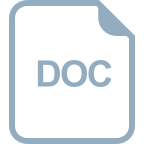
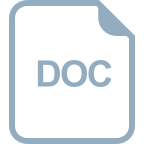
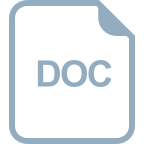