写一个矩阵类CMatrix,基本要求如下:1.实现构造、拷贝构造与析构函数;2.支持任意大小的矩阵,行数和列数可以动态设置(不用修改代码);3.重载操作符*,实现矩阵相乘;4.重载操作符=,实现矩阵赋值;5.重载操作符++和--,分别实现矩阵元素同时+1和-1;在main函数中对以上功能进行调用
时间: 2024-03-06 17:52:08 浏览: 61
好的,我会解答您的问题。下面是一个简单的实现:
```c++
#include<iostream>
using namespace std;
class CMatrix{
private:
int rows, cols;
int** data;
public:
CMatrix(int m=0, int n=0): rows(m), cols(n){
data = new int*[rows];
for(int i=0; i<rows; i++){
data[i] = new int[cols];
for(int j=0; j<cols; j++){
data[i][j] = 0;
}
}
}
CMatrix(const CMatrix& mat){
this->rows = mat.rows;
this->cols = mat.cols;
this->data = new int*[rows];
for(int i=0; i<rows; i++){
data[i] = new int[cols];
for(int j=0; j<cols; j++){
data[i][j] = mat.data[i][j];
}
}
}
~CMatrix(){
for(int i=0; i<rows; i++){
delete[] data[i];
}
delete[] data;
}
CMatrix operator*(const CMatrix& mat){
CMatrix res(rows, mat.cols);
for(int i=0; i<res.rows; i++){
for(int j=0; j<res.cols; j++){
for(int k=0; k<cols; k++){
res.data[i][j] += data[i][k] * mat.data[k][j];
}
}
}
return res;
}
CMatrix& operator=(const CMatrix& mat){
if(this == &mat) return *this;
for(int i=0; i<rows; i++){
delete[] data[i];
}
delete[] data;
this->rows = mat.rows;
this->cols = mat.cols;
this->data = new int*[rows];
for(int i=0; i<rows; i++){
data[i] = new int[cols];
for(int j=0; j<cols; j++){
data[i][j] = mat.data[i][j];
}
}
return *this;
}
CMatrix& operator++(){
for(int i=0; i<rows; i++){
for(int j=0; j<cols; j++){
data[i][j]++;
}
}
return *this;
}
CMatrix operator++(int){
CMatrix temp(*this);
operator++();
return temp;
}
CMatrix& operator--(){
for(int i=0; i<rows; i++){
for(int j=0; j<cols; j++){
data[i][j]--;
}
}
return *this;
}
CMatrix operator--(int){
CMatrix temp(*this);
operator--();
return temp;
}
friend ostream& operator<<(ostream& os, const CMatrix& mat){
for(int i=0; i<mat.rows; i++){
for(int j=0; j<mat.cols; j++){
os << mat.data[i][j] << " ";
}
os << endl;
}
return os;
}
};
int main(){
CMatrix mat1(2, 3), mat2(3, 2);
for(int i=0; i<2; i++){
for(int j=0; j<3; j++){
mat1.data[i][j] = i+j;
}
}
for(int i=0; i<3; i++){
for(int j=0; j<2; j++){
mat2.data[i][j] = i*j;
}
}
cout << "mat1:" << endl << mat1;
cout << "mat2:" << endl << mat2;
CMatrix mat3 = mat1 * mat2;
cout << "mat3:" << endl << mat3;
mat1++;
cout << "mat1++:" << endl << mat1;
CMatrix mat4 = mat2--;
cout << "mat2--:" << endl << mat2;
cout << "mat4:" << endl << mat4;
return 0;
}
```
上述代码定义了一个矩阵类`CMatrix`,其构造函数可以初始化一个指定大小的矩阵,也有拷贝构造函数和析构函数。该类还重载了操作符`*`,实现了矩阵乘法;操作符`=`,实现了矩阵赋值;操作符`++`和`--`,分别实现了矩阵元素同时+1和-1。在main函数中进行了上述功能的调用。
阅读全文
相关推荐












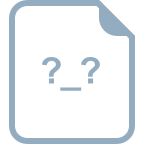
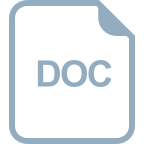
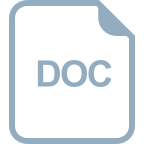

