写一个矩阵类cMatrix,基本要求如下:实现构造、拷贝构造与析构函数;支持任意大小的矩阵:行数和列数可以动态设置(不用修改代码)重载操作符*,实现矩阵相乘;重载操作符=,实现矩阵赋值;重载操作符++和--,分别实现矩阵元素同时+1和-1在main函数中对以上功能进行调用
时间: 2024-03-14 15:45:12 浏览: 18
好的,以下是cMatrix类的实现,包含所需的功能:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class cMatrix {
private:
int rows; // 矩阵行数
int cols; // 矩阵列数
int **data; // 矩阵数据
public:
// 构造函数
cMatrix(int r, int c) : rows(r), cols(c) {
// 分配内存
data = new int *[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
// 初始化为0
memset(data[i], 0, sizeof(int) * cols);
}
}
// 拷贝构造函数
cMatrix(const cMatrix &m) : rows(m.rows), cols(m.cols) {
// 分配内存
data = new int *[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
// 复制数据
memcpy(data[i], m.data[i], sizeof(int) * cols);
}
}
// 析构函数
~cMatrix() {
// 释放内存
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
}
// 矩阵相乘
cMatrix operator*(const cMatrix &m) const {
// 检查矩阵维度是否匹配
if (cols != m.rows) {
cerr << "Error: Matrix dimensions do not match!" << endl;
exit(1);
}
cMatrix result(rows, m.cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < m.cols; j++) {
for (int k = 0; k < cols; k++) {
result.data[i][j] += data[i][k] * m.data[k][j];
}
}
}
return result;
}
// 矩阵赋值
cMatrix &operator=(const cMatrix &m) {
// 检查是否自我赋值
if (this == &m) {
return *this;
}
// 释放原有内存
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
// 分配新内存
rows = m.rows;
cols = m.cols;
data = new int *[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
// 复制数据
memcpy(data[i], m.data[i], sizeof(int) * cols);
}
return *this;
}
// 矩阵元素同时+1
cMatrix operator++() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
data[i][j]++;
}
}
return *this;
}
// 矩阵元素同时-1
cMatrix operator--() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
data[i][j]--;
}
}
return *this;
}
// 输出矩阵
friend ostream &operator<<(ostream &out, const cMatrix &m) {
for (int i = 0; i < m.rows; i++) {
for (int j = 0; j < m.cols; j++) {
out << m.data[i][j] << " ";
}
out << endl;
}
return out;
}
};
```
以下是在main函数中对以上功能进行调用的示例代码:
```c++
int main() {
cMatrix m1(3, 2);
cMatrix m2(2, 4);
// 设置矩阵数据
int cnt = 0;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 2; j++) {
m1[i][j] = cnt++;
}
}
cnt = 0;
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 4; j++) {
m2[i][j] = cnt++;
}
}
// 输出矩阵
cout << "m1:" << endl << m1 << endl;
cout << "m2:" << endl << m2 << endl;
// 矩阵相乘
cMatrix m3 = m1 * m2;
cout << "m1 * m2:" << endl << m3 << endl;
// 矩阵赋值
cMatrix m4(2, 3);
m4 = m3;
cout << "m4:" << endl << m4 << endl;
// 矩阵元素同时+1
++m4;
cout << "++m4:" << endl << m4 << endl;
// 矩阵元素同时-1
--m4;
cout << "--m4:" << endl << m4 << endl;
return 0;
}
```
相关推荐
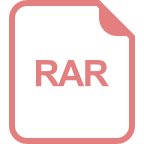













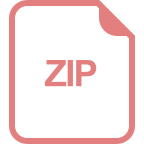