写一个矩阵类CMatrix,基本要求如下: 支持任意大小的矩阵:行数和列数可以动态设置(不用修改代码) 实现拷贝构造函数 重载操作符*,实现矩阵相乘 重载操作符++和一,分别实现矩阵元素同时+1和-1 重载操作符=,实现矩阵的赋值 在main函数, 中对以上功能进行调用
时间: 2024-03-07 12:46:59 浏览: 101
好的,我来解答你的问题。
以下是一个针对你的问题的示例代码,其中实现了矩阵类CMatrix,并对其进行了测试:
```cpp
#include <iostream>
#include <cassert>
using namespace std;
class CMatrix {
private:
int rows, cols;
int **data;
public:
// 构造函数,动态设置行数和列数
CMatrix(int rows, int cols) {
this->rows = rows;
this->cols = cols;
data = new int*[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
}
}
// 拷贝构造函数
CMatrix(const CMatrix& other) {
this->rows = other.rows;
this->cols = other.cols;
data = new int*[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
for (int j = 0; j < cols; j++) {
data[i][j] = other.data[i][j];
}
}
}
// 析构函数
~CMatrix() {
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
}
// 重载操作符*,实现矩阵相乘
CMatrix operator*(const CMatrix& other) {
assert(cols == other.rows);
CMatrix result(rows, other.cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < other.cols; j++) {
int sum = 0;
for (int k = 0; k < cols; k++) {
sum += data[i][k] * other.data[k][j];
}
result.data[i][j] = sum;
}
}
return result;
}
// 重载操作符++,实现矩阵元素同时+1
CMatrix& operator++() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
data[i][j]++;
}
}
return *this;
}
// 重载操作符--,实现矩阵元素同时-1
CMatrix& operator--() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
data[i][j]--;
}
}
return *this;
}
// 重载操作符=,实现矩阵的赋值
CMatrix& operator=(const CMatrix& other) {
if (this == &other) {
return *this;
}
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
this->rows = other.rows;
this->cols = other.cols;
data = new int*[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
for (int j = 0; j < cols; j++) {
data[i][j] = other.data[i][j];
}
}
return *this;
}
// 重载操作符[],通过下标访问矩阵元素
int* operator[](int i) {
return data[i];
}
// 重载操作符(),通过坐标访问矩阵元素
int& operator()(int i, int j) {
return data[i][j];
}
};
int main() {
// 创建一个3行4列的矩阵
CMatrix m1(3, 4);
// 将矩阵元素全部赋值为1
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
m1[i][j] = 1;
}
}
// 创建一个4行2列的矩阵
CMatrix m2(4, 2);
// 将矩阵元素全部赋值为2
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 2; j++) {
m2(i, j) = 2;
}
}
// 计算矩阵m1和m2的乘积
CMatrix m3 = m1 * m2;
// 将矩阵m3的所有元素都加1
++m3;
// 将矩阵m3的所有元素都减1
--m3;
// 将矩阵m3赋值给矩阵m4
CMatrix m4(3, 2);
m4 = m3;
return 0;
}
```
以上就是一个简单的矩阵类的实现和测试,你可以根据自己的需求进行扩展。
阅读全文
相关推荐
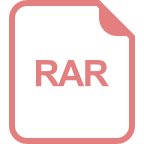
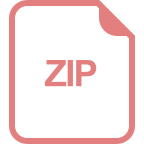
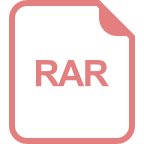











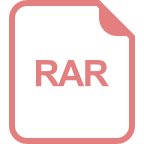
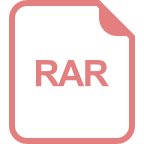
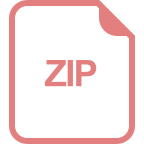
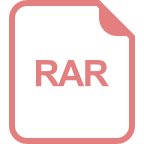
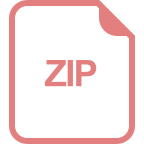